Python之dict(或对象)与json之间的互相转化
在Python语言中,json数据与dict字典以及对象之间的转化,是必不可少的操作。
在Python中自带json库。通过 import json
导入。
在json模块有2个方法,
- loads():将json数据转化成dict数据
- dumps():将dict数据转化成json数据
- load():读取json文件数据,转成dict数据
- dump():将dict数据转化成json数据后写入json文件
下面是具体的示例:
dict字典转json数据
# -*- coding: utf-8 -*-
import json
# dict字典转json数据
def dict_to_json():
dict = {}
dict['name'] = 'Joe.Ye'
dict['age'] = 20
dict['sex'] = 'male'
print(dict) # 输出: {'name': 'many', 'age': 10, 'sex': 'male'}
j = json.dumps(dict)
print(j) # 输出: {"name": "many", "age": 10, "sex": "male"}
# 对象转json数据
def obj_to_json():
stu = Student(1, 'Joe.Ye', 28, 'male', '18800000000', 'test@test.com')
print(type(stu)) # <class 'json_test.student.Student'>
stu = stu.__dict__ # 将对象转成dict字典
print(type(stu)) # <class 'dict'>
print(stu) # {'name': 'Joe.Ye', 'age': 28, 'sex': 'male', 'phone': '18800000000', 'email': 'test@test.com', 'id': 1}
j = json.dumps(obj=stu)
print(j) # {"name": "Joe.Ye", "age": 28, "sex": "male", "phone": "18800000000", "email": "test@test.com", "id": 1}
# json数据转成dict字典
def json_to_dict():
j = '{"id": 1, "name": "Joe.Ye", "age": 28, "sex": "male", "phone": "18800000000", "email": "test@test.com"}'
dict = json.loads(s=j)
print(dict) # {u'name': u'Joe.Ye', u'age': 28, u'sex': u'male', u'phone': u'18800000000', u'email': u'test@test.com', u'id': 1}
# json数据转成对象
def json_to_obj():
j = '{"id": 1, "name": "Joe.Ye", "age": 28, "sex": "male", "phone": "18800000000", "email": "test@test.com"}'
dict = json.loads(s=j)
stu = Student()
stu.__dict__ = dict
print('id: ' + str(stu.id) + ', name: ' + stu.name + ', age: ' + str(stu.age) + ', sex: ' + str(stu.sex) +
', phone: ' + stu.phone + ', email: ' + stu.email) # id: 1, name: Joe.Ye, age: 28, sex: male, phone: 18800000000, email: test@test.com
class Student():
# Python通过参数默认值方式实现构造函数重载
def __init__(self, id=0, name='', age=0, sex='', phone='', email=''):
self.id = id
self.name = name
self.age = age
self.sex = sex
self.phone = phone
self.email = email
# dump()方法的使用
def dict_to_json_write_file():
dict = {}
dict['name'] = 'Joe.Ye'
dict['age'] = 10
dict['sex'] = 'male'
print(dict) # {'age': 10, 'name': 'Joe.Ye', 'sex': 'male'}
with open('test.json', 'w') as f:
json.dump(dict, f) # 会在目录下生成一个test.json的文件,文件内容是dict数据转成的json数据
# load()方法的使用
def json_file_to_dict():
with open('test.json', 'r') as f:
dict = json.load(fp=f)
print(dict) # {u'age': 10, u'name': u'Joe.Ye', u'sex': u'male'}
if __name__ == '__main__':
dict_to_json()
obj_to_json()
json_to_dict()
json_to_obj()
dict_to_json_write_file()
json_file_to_dict()
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/22/mutual-conversion-between-dict-or-object-and-json-in-python/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

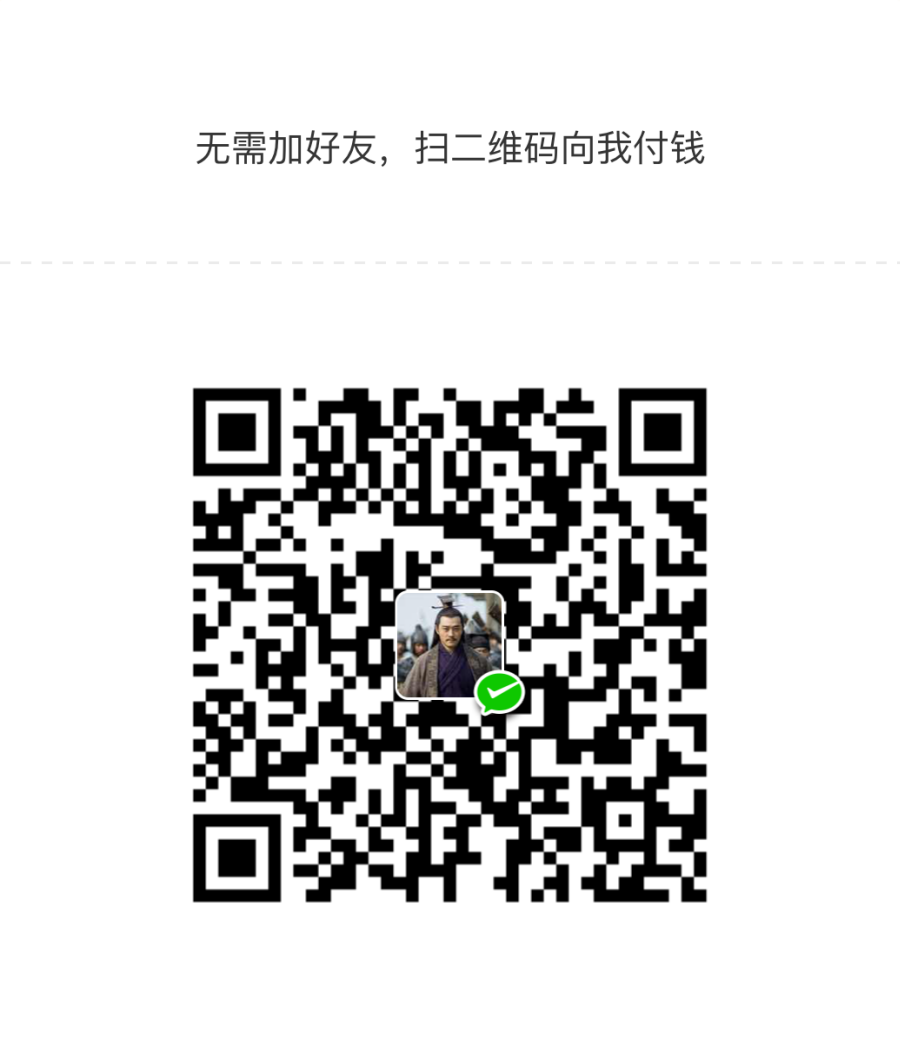
Python之dict(或对象)与json之间的互相转化
在Python语言中,json数据与dict字典以及对象之间的转化,是必不可少的操作。
在Python中自带json库。通过 import json 导入。
在json模块有2个方法,
loads……

文章目录
关闭
共有 0 条评论