Python使用POP3读取邮箱中的邮件,含文本及附件
读取邮件列表
import poplib
import email
from email.parser import Parser
def receive_email_pop3(address, password):
# 防止报错: poplib.error_proto: line too long
poplib._MAXLINE = 1024 * 1024
pop3 = poplib.POP3('imap.exmail.qq.com', 110)
# pop3 = poplib.POP3_SSL('imap.exmail.qq.com', 995)
pop3.user(address)
pop3.pass_(password)
# 获取一些统计信息
emailMsgNum, emailSize = pop3.stat()
print 'Email number is %d and size is %d' % (emailMsgNum, emailSize)
# 遍历邮件,并打印出每封邮件的标题
for i in range(emailMsgNum):
for piece in pop3.retr(i + 1)[1]:
# print piece
if piece.startswith('Subject'):
print piece
break
# Get messages from server:
# 获得邮件
messages = [pop3.retr(i) for i in range(1, len(pop3.list()[1]) + 1)]
# print messages
# Concat message pieces:
messages = ["\n".join(msg[1]) for msg in messages]
# print messages
# Parse message intom an email object:
# 分析
# 'LazyImporter' object is not callable
# https://stackoverflow.com/questions/34348069/typeerror-lazyimporter-object-is-not-callable
# messages = [parser.Parser().parsestr(mssg) for mssg in messages]
messages = [Parser().parsestr(msg) for msg in messages]
i = 0
for index in range(0, len(messages)):
message = messages[index]
i = i + 1
subject = message.get('subject')
print subject
# h = email.Header(subject)
# dh = email.Header.decode_header(h)
# subject = unicode(dh[0][0], dh[0][1]).encode('utf8')
mailName = "mail%d.%s" % (i, subject)
f = open('%d.log' % (i), 'w')
print >> f, "Date: ", message["Date"]
print >> f, "From: ", email.utils.parseaddr(message.get('from'))[1]
print >> f, "To: ", email.utils.parseaddr(message.get('to'))[1]
print >> f, "Subject: ", subject
print >> f, "Data: "
j = 0
for part in message.walk():
j = j + 1
fileName = part.get_filename()
contentType = part.get_content_type()
contentCharset = part.get_content_charset()
# 保存附件
if fileName:
data = part.get_payload(decode=True)
h = email.Header.Header(fileName)
dh = email.Header.decode_header(h)
fname = dh[0][0]
encodeStr = dh[0][1]
if encodeStr != None:
fname = fname.decode(encodeStr, contentCharset)
# end if
fEx = open("%s" % (fname), 'wb')
fEx.write(data)
fEx.close()
elif contentType == 'text/plain' or contentType == 'text/html': # or contentType == 'text/html':
# 保存正文
data = part.get_payload(decode=True)
email_content = str(data).decode(contentCharset).encode("utf-8")
print >> f, data
# end if
# end for
f.close()
# end for
pop3.quit()
读取最新邮件
import poplib
import email
from email.parser import Parser
class EmailLib():
def __init__(self):
self.pop3_server = 'imap.exmail.qq.com'
self.pop3_port = 110
self.pop3_ssl = False
pass
def receive_email_pop3(self, address, password):
# 防止报错: poplib.error_proto: line too long
poplib._MAXLINE = 1024 * 1024
if self.pop3_ssl:
pop3 = poplib.POP3_SSL(self.pop3_server, self.pop3_port)
else:
pop3 = poplib.POP3(self.pop3_server, self.pop3_port)
pop3.user(address)
pop3.pass_(password)
# 获取一些统计信息
emailMsgNum, emailSize = pop3.stat()
print 'Email number is %d and size is %d' % (emailMsgNum, emailSize)
# Get messages from server:
# 获得邮件
messages = [pop3.retr(i) for i in range(1, len(pop3.list()[1]) + 1)]
# Concat message pieces:
messages = ["\n".join(msg[1]) for msg in messages]
messages = [Parser().parsestr(msg) for msg in messages]
# 遍历邮件,并打印出每封邮件的标题
email_from = ''
email_to = ''
email_subject = ''
email_content = ''
email_date = ''
for i in range(emailMsgNum-1, -1, -1):
print '正在查询第 %d 封邮件' % (emailMsgNum-i)
message = messages[i]
email_subject = message.get('subject')
email_date = message["Date"]
email_from = email.utils.parseaddr(message.get('from'))[1]
email_to = email.utils.parseaddr(message.get('to'))[1]
for part in message.walk():
contentType = part.get_content_type()
contentCharset = part.get_content_charset()
if contentType == 'text/plain' or contentType == 'text/html':
# 保存正文
data = part.get_payload(decode=True)
email_content = str(data).decode(contentCharset).encode("utf-8")
# end if
# end for
if 'Apollo@lianlianpayglobal.com' in email_from:
break
# print email_from
# print email_to
# print email_subject
# print email_date
# print email_content
pop3.quit()
ret_email = {}
ret_email['from'] = email_from
ret_email['to'] = email_to
ret_email['subject'] = email_subject
ret_email['date'] = email_date
ret_email['content'] = email_content
print '邮件主题:' + email_subject
print '邮件内容:' + email_content
return ret_email
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/22/python-use-pop3-to-read-emails-in-mailbox-including-text-and-attachments/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

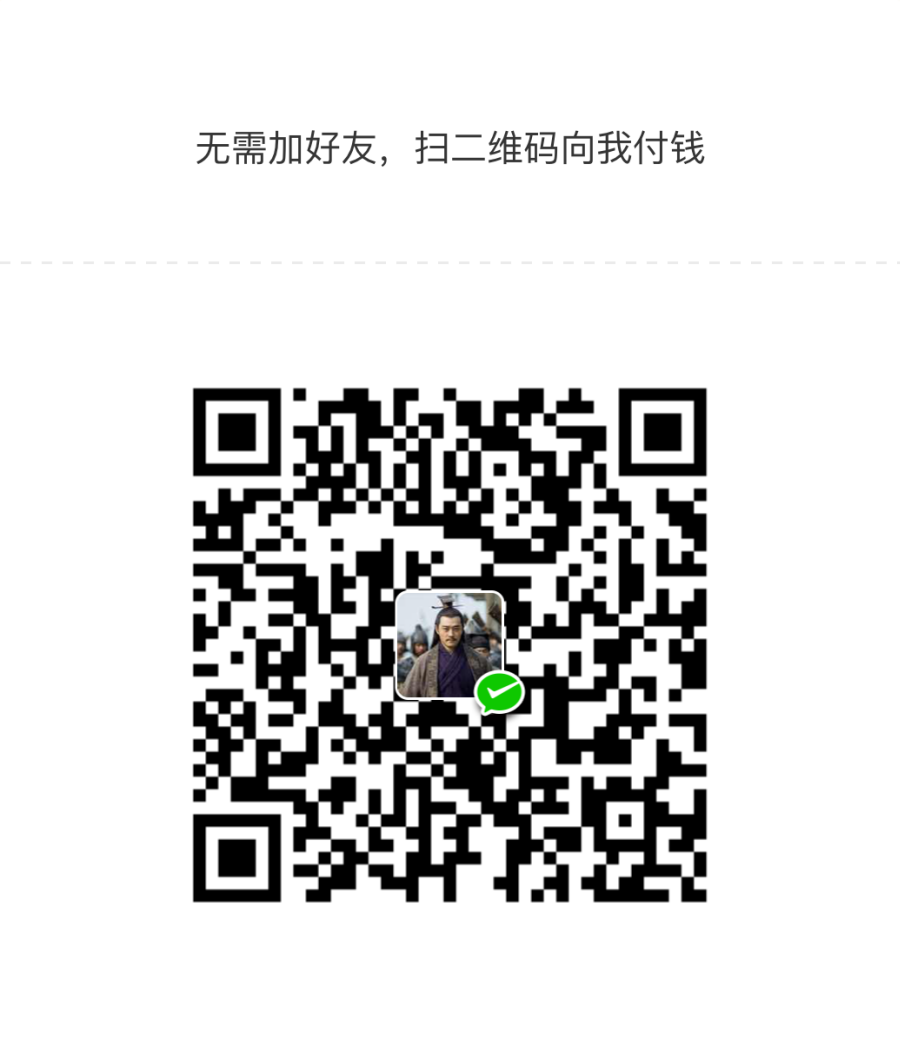
Python使用POP3读取邮箱中的邮件,含文本及附件
读取邮件列表
import poplib
import email
from email.parser import Parser
def receive_email_pop3(address, password):
# 防止报错: poplib.error_pr……

文章目录
关闭
共有 0 条评论