Android NDK基础20:C++_类大小_友元函数_运算符重载
类大小
//类的大小
class A {
public:
int i;
int j;
int k;
static int m;
};
class B {
public:
int i;
int j;
int k;
void printf() {
cout << "打印" << endl;
}
};
void main() {
cout << sizeof(A) << endl; //12
cout << sizeof(B) << endl; //12
//C/C++ 内存分区:栈、堆、全局(静态、全局)、常量区(字符串)、程序代码区
//普通属性与结构体相同的内存布局
//JVM 内存分区:
//JVM Stack(基本数据类型、对象引用)
//Native Method Stack(本地方法栈)
//方法区
system("pause");
}
友元函数
//友元函数
class A {
//友元函数
friend void modify_i(A *p, int a);
private:
int i;
public:
A(int i) {
this->i = i;
}
void print() {
cout << i << endl;
}
};
//友元函数的实现,在友元函数中可以访问私有的属性
void modify_i(A *p, int a) {
p->i = a;
}
void main() {
A* a = new A(10);
a->print();
modify_i(a, 20);
a->print();
system("pause");
}
//友元类
class A {
//友元类
friend class B;
private:
int i;
public:
A(int i) {
this->i = i;
}
void print() {
cout << i << endl;
}
};
class B {
public:
//B这个友元类可以访问A类的任何成员
void accessAny() {
a.i = 30;
}
private:
A a;
};
运算符重载
//运算符重载
class Point {
public:
int x;
int y;
public:
Point(int x = 0, int y = 0) {
this->x = x;
this->y = y;
}
void print() {
cout << x << "," << y << endl;
}
};
//重载+号
Point operator+(Point &p1, Point &p2) {
Point p(p1.x + p2.x, p1.y + p2.y);
return p;
}
//重载-号
Point operator-(Point &p1, Point &p2) {
Point p(p1.x - p2.x, p1.y - p2.y);
return p;
}
void main() {
Point p1(10, 20);
Point p2(20, 10);
Point p3 = p1 + p2;
p3.print();
system("pause");
}
//成员函数,运算符重载
class Point {
public:
int x;
int y;
public:
Point(int x = 0, int y = 0) {
this->x = x;
this->y = y;
}
//成员函数,运算符重载
Point operator+(Point &p2) {
Point tmp(this->x + p2.x, this->y + p2.y);
return tmp;
}
void print() {
cout << x << "," << y << endl;
}
};
void main() {
Point p1(10, 20);
Point p2(20, 10);
//运算符的重载,本质还是函数调用
//p1.operator+(p2)
Point p3 = p1 + p2;
p3.print();
system("pause");
}
//当属性私有时,通过友元函数完成运算符重载
class Point {
friend Point operator+(Point &p1, Point &p2);
private:
int x;
int y;
public:
Point(int x = 0, int y = 0) {
this->x = x;
this->y = y;
}
void print() {
cout << x << "," << y << endl;
}
};
Point operator+(Point &p1, Point &p2) {
Point p(p1.x + p2.x, p1.y + p2.y);
return p;
}
void main() {
Point p1(10, 20);
Point p2(20, 10);
//运算符的重载,本质还是函数调用
//p1.operator+(p2)
Point p3 = p1 + p2;
p3.print();
system("pause");
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/25/android-ndk-basic-cpp-class-size-friend-function-operator-overloading/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

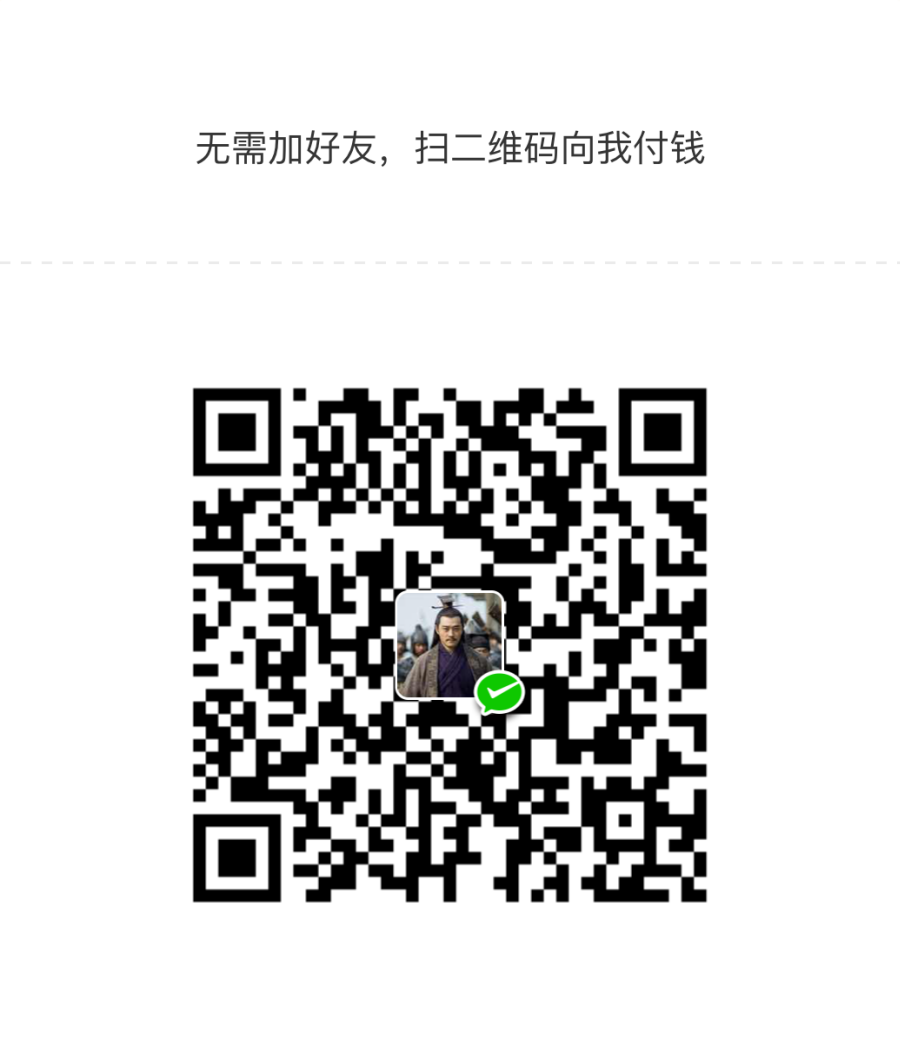
Android NDK基础20:C++_类大小_友元函数_运算符重载
类大小
//类的大小
class A {
public:
int i;
int j;
int k;
static int m;
};
class B {
public:
int i;
int j;
int k;
v……

文章目录
关闭
共有 0 条评论