Java实现GZIP压缩与解压缩
Gzip压缩
public static byte[] compress(String str, String charset) {
if (str == null || str.length() == 0) {
return null;
}
ByteArrayOutputStream out = new ByteArrayOutputStream();
GZIPOutputStream gzip;
try {
gzip = new GZIPOutputStream(out);
gzip.write(str.getBytes(charset));
gzip.close();
} catch (Exception e) {
e.printStackTrace();
}
return out.toByteArray();
}
Gzip解压缩
public static byte[] uncompress(byte[] bytes) {
if (bytes == null || bytes.length == 0) {
return null;
}
ByteArrayOutputStream out = new ByteArrayOutputStream();
ByteArrayInputStream in = new ByteArrayInputStream(bytes);
try {
GZIPInputStream unGzip = new GZIPInputStream(in);
byte[] buffer = new byte[256];
int n;
while ((n = unGzip.read(buffer)) >= 0) {
out.write(buffer, 0, n);
}
} catch (Exception e) {
e.printStackTrace();
}
return out.toByteArray();
}
工具代码集合
public class GzipUtils {
public static byte[] compress(String str, String charset) {
if (str == null || str.length() == 0) {
return null;
}
ByteArrayOutputStream out = new ByteArrayOutputStream();
GZIPOutputStream gzip;
try {
gzip = new GZIPOutputStream(out);
gzip.write(str.getBytes(charset));
gzip.close();
} catch (Exception e) {
e.printStackTrace();
}
return out.toByteArray();
}
public static byte[] compress(String str) throws IOException {
return compress(str, StandardCharsets.UTF_8.name());
}
public static byte[] uncompress(byte[] bytes) {
if (bytes == null || bytes.length == 0) {
return null;
}
ByteArrayOutputStream out = new ByteArrayOutputStream();
ByteArrayInputStream in = new ByteArrayInputStream(bytes);
try {
GZIPInputStream unGzip = new GZIPInputStream(in);
byte[] buffer = new byte[256];
int n;
while ((n = unGzip.read(buffer)) >= 0) {
out.write(buffer, 0, n);
}
} catch (Exception e) {
e.printStackTrace();
}
return out.toByteArray();
}
public static byte[] uncompress(String str, String charset) throws IOException {
return uncompress(str.getBytes(charset));
}
public static byte[] uncompress(String str) throws IOException {
return uncompress(str.getBytes(StandardCharsets.UTF_8.name()));
}
public static String uncompressToString(byte[] bytes, String charset) {
if (bytes == null || bytes.length == 0) {
return null;
}
ByteArrayOutputStream out = new ByteArrayOutputStream();
ByteArrayInputStream in = new ByteArrayInputStream(bytes);
try {
GZIPInputStream unGzip = new GZIPInputStream(in);
byte[] buffer = new byte[256];
int n;
while ((n = unGzip.read(buffer)) >= 0) {
out.write(buffer, 0, n);
}
return out.toString(charset);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public static String uncompressToString(byte[] bytes) {
return uncompressToString(bytes, StandardCharsets.UTF_8.name());
}
public static String uncompressToString(String str, String inputCharset, String outputCharset) throws IOException {
return new String(uncompress(str, inputCharset), outputCharset);
}
public static String uncompressToString(String str) throws IOException {
return new String(uncompress(str, StandardCharsets.ISO_8859_1.name()), StandardCharsets.UTF_8.name());
}
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/25/java-implement-gzip-compression-and-decompression/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

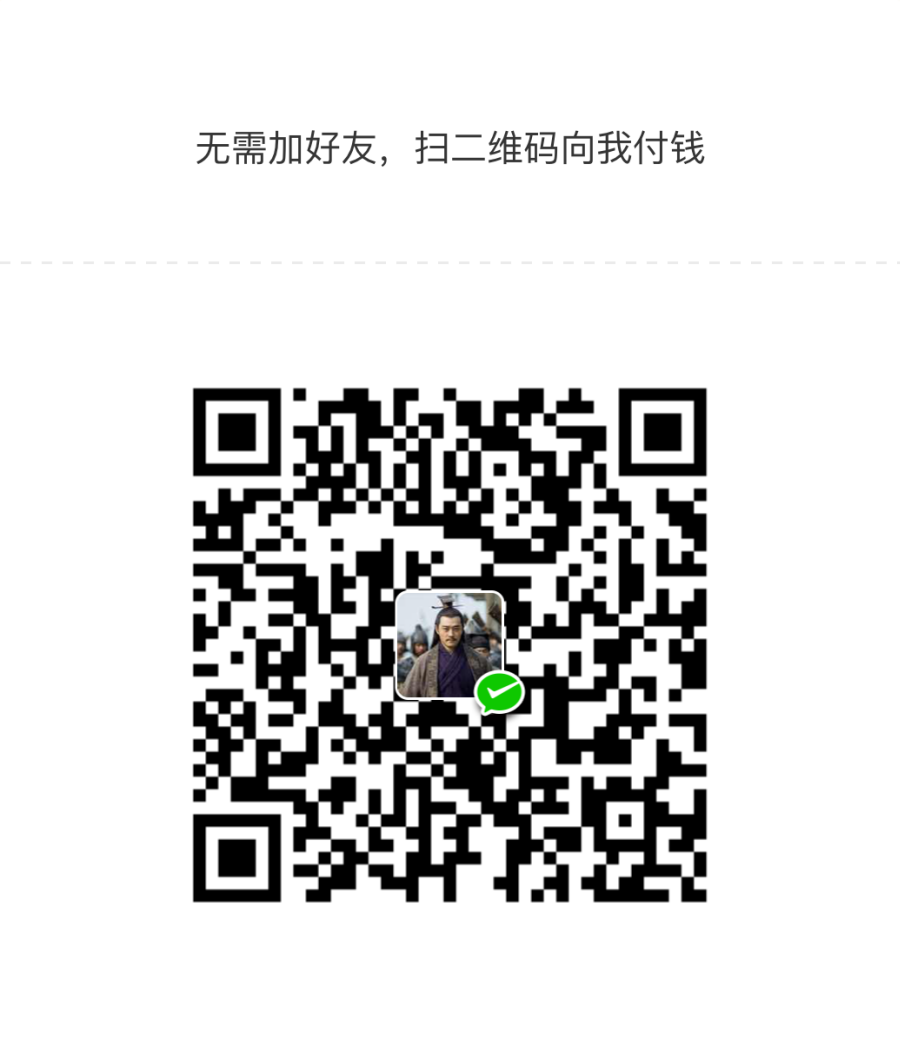
Java实现GZIP压缩与解压缩
Gzip压缩
public static byte[] compress(String str, String charset) {
if (str == null || str.length() == 0) {
return null;
}
Byte……

文章目录
关闭
共有 0 条评论