Swift UI – 使用UIDatePicker实现倒计时功能
注:代码已升级至Swift4
如果使用UIDatePicker时将模式设置为CountDownTimer,即可让该控件作为倒计时器来使用。
代码示例
import UIKit
class ViewController: UIViewController {
var ctimer:UIDatePicker!
var btnStart:UIButton!
var leftTime:Int = 180
var alertController:UIAlertController!
var timer:Timer!
override func viewDidLoad() {
super.viewDidLoad()
ctimer = UIDatePicker(frame:CGRect(x:0, y:120, width:200, height:200))
self.ctimer.datePickerMode = UIDatePickerMode.countDownTimer
//必须为60的整数倍,比如设置为100,值自动变为60
self.ctimer.countDownDuration = TimeInterval(leftTime)
ctimer.addTarget(self, action: #selector(ViewController.timerChanged),
for: .valueChanged)
self.view.addSubview(ctimer)
btnStart = UIButton(type: .system)
btnStart.frame = CGRect(x:100, y:400, width:100, height:100);
btnStart.setTitleColor(UIColor.red, for: .normal)
btnStart.setTitleColor(UIColor.green, for:.disabled)
btnStart.setTitle("开始", for:.normal)
btnStart.setTitle("倒计时中", for:.disabled)
btnStart.clipsToBounds = true
btnStart.layer.cornerRadius = 5
btnStart.addTarget(self, action:#selector(ViewController.startClicked),
for:.touchUpInside)
self.view.addSubview(btnStart)
}
@objc func timerChanged() {
print("您选择倒计时间为:\(self.ctimer.countDownDuration)")
}
/**
* 开始倒计时按钮点击
*/
@objc func startClicked(sender:UIButton) {
self.btnStart.isEnabled = false
// 获取该倒计时器的剩余时间
leftTime = Int(self.ctimer.countDownDuration);
// 禁用UIDatePicker控件和按钮
self.ctimer.isEnabled = false
// 创建一个UIAlertController对象(警告框),并确认,倒计时开始
alertController = UIAlertController(title: "系统提示",
message: "倒计时开始,还有 \(leftTime) 秒...",
preferredStyle: .alert)
let cancelAction = UIAlertAction(title: "确定", style: .cancel, handler: nil)
alertController.addAction(cancelAction)
// 显示UIAlertController组件
self.present(alertController, animated: true, completion: nil)
// 启用计时器,控制每秒执行一次tickDown方法
timer = Timer.scheduledTimer(timeInterval: TimeInterval(1), target:self,
selector:#selector(ViewController.tickDown),
userInfo:nil, repeats:true)
}
/**
* 计时器每秒触发事件
*/
@objc func tickDown() {
alertController.message = "倒计时开始,还有 \(leftTime) 秒..."
// 将剩余时间减少1秒
leftTime -= 1;
// 修改UIDatePicker的剩余时间
self.ctimer.countDownDuration = TimeInterval(leftTime);
print(leftTime)
// 如果剩余时间小于等于0
if (leftTime <= 0) {
// 取消定时器
timer.invalidate();
// 启用UIDatePicker控件和按钮
self.ctimer.isEnabled = true;
self.btnStart.isEnabled = true;
alertController.message = "时间到!"
}
}
}
问题解决
上面的代码其实还是有个小Bug的
-
问题描述:代码中给时间控件添加了个 ValueChanged 事件监听响应,目的是想每次选择的时间改变时都会触发打印出时间。但运行会发现,第一次拨动表盘不触发,后面再改变值才会触发。
-
解决办法:这个是iOS的bug,我们把设置初始时间代码
//必须为 60 的整数倍,比如设置为100,值自动变为 60
self.ctimer.countDownDuration = TimeInterval(leftTime)
修改成
DispatchQueue.main.async {
self.ctimer.countDownDuration = TimeInterval(self.leftTime)
}
如果我们不需要关心值改变事件的话,直接用原来的赋值方法即可
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/25/swift-ui-implement-countdown-function-using-uidatepicker/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

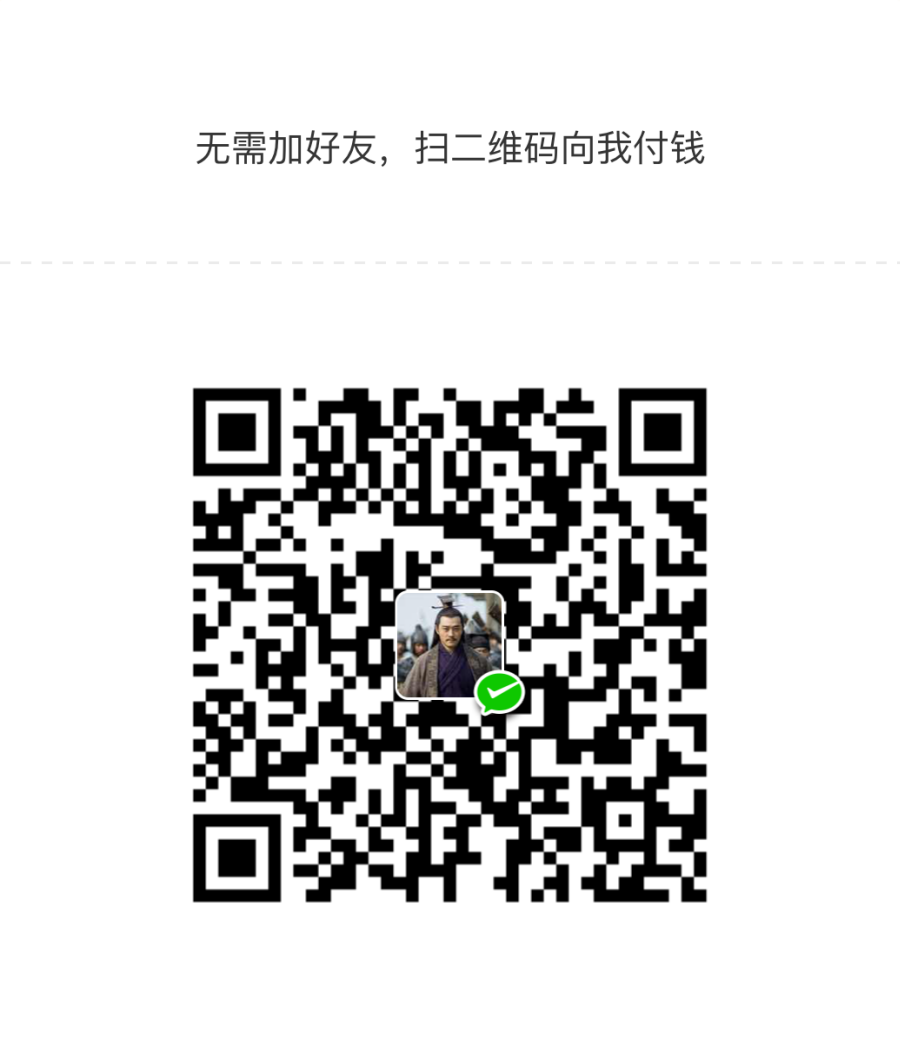
Swift UI – 使用UIDatePicker实现倒计时功能
注:代码已升级至Swift4
如果使用UIDatePicker时将模式设置为CountDownTimer,即可让该控件作为倒计时器来使用。
代码示例
import UIKit
class ViewContro……

文章目录
关闭
共有 0 条评论