Swift UI – 多行文本输入框(UITextView)
注:代码已升级至Swift4
多行文本控件的创建
文本输入框支持的样式:
let textview = UITextView(frame:CGRect(x:10, y:100, width:200, height:100))
textview.layer.borderWidth = 1 //边框粗细
textview.layer.borderColor = UIColor.gray.cgColor //边框颜色
self.view.addSubview(textview)
文本内容
//设置显示内容
textView.text ="Welcome to AppBlog.CN"
是否可滚动
//是否可以滚动
textView.scrollEnabled = true
文本高度
//自适应高度
textView.autoresizingMask = UIViewAutoresizing.FlexibleHeight
//获取内容整体高度
var height:CGFloat = textView.contentSize.height
是否可编辑
textview.isEditable = false
内容是否可选
textview.isSelectable = false
文本选中
//选中一段文本
textView.becomeFirstResponder()
textView.selectedRange = NSMakeRange(30, 10)
字体及颜色
//背景颜色设置
textView.backgroundColor = UIColor.grayColor()
//设置textview里面的字体颜色
textView.textColor = UIColor.greenColor()
//设置文本字体
textView.font = UIFont.systemFontOfSize(18); //使用系统默认字体,指定14号字号
textView.font = UIFont(name: "Helvetica-Bold", size: 18) //指定字体,指定字号
设置对齐方式
//文本对齐方式
textView.textAlignment = .left
//其中UITextField的文本的对齐方式
public enum NSTextAlignment : Int {
case left // Visually left aligned
case center // Visually centered
case right // Visually right aligned
/* !TARGET_OS_IPHONE */
// Visually right aligned
// Visually centered
case justified // Fully-justified. The last line in a paragraph is natural-aligned.
case natural // Indicates the default alignment for script
}
设置圆角
//文本视图设置圆角
textView.layer.cornerRadius = 20
textView.layer.masksToBounds = true
设置富文本
//设置富文本
var attributeString:NSMutableAttributedString=NSMutableAttributedString(string: "经常听到,押金不退,晚一天交房租,被讹了。网上报价不真实?经常被忽悠")
//设置字体颜色
attributeString.addAttribute(NSForegroundColorAttributeName, value: UIColor.greenColor(), range: NSMakeRange(0, attributeString.length))
//文本所有字符字体HelveticaNeue-Bold,16号
attributeString.addAttribute(NSFontAttributeName, value: UIFont(name: "HelveticaNeue-Bold", size: 16)!, range: NSMakeRange(0, attributeString.length))
//文本0开始5个字符字体HelveticaNeue-Bold,16号
attributeString.addAttribute(NSFontAttributeName, value: UIFont(name: "HelveticaNeue-Bold", size: 26)!, range: NSMakeRange(0, 5))
//设置字体颜色
attributeString.addAttribute(NSForegroundColorAttributeName, value: UIColor.whiteColor(), range: NSMakeRange(0, 3))
//设置文字背景颜色
attributeString.addAttribute(NSBackgroundColorAttributeName, value: UIColor.orangeColor(), range: NSMakeRange(3, 3))
//赋值富文本
textView.attributedText = attributeString
给文字中的电话号码和网址自动加链接
textview.dataDetectorTypes = [] //都不加链接
textview.dataDetectorTypes = UIDataDetectorTypes.phoneNumber //只有电话加链接
textview.dataDetectorTypes = UIDataDetectorTypes.link //只有网址加链接
textview.dataDetectorTypes = UIDataDetectorTypes.all //电话和网址都加
//是否允许点击链接和附件
textView.isSelectable = true
返回键类型
//返回键的类型
textView.returnKeyType = UIReturnKeyType.Done
//键盘的返回键类型
public enum UIReturnKeyType : Int {
case `default`
case go
case google
case join
case next
case route
case search
case send
case yahoo
case done
case emergencyCall
@available(iOS 9.0, *)
case `continue`
}
键盘类型
//键盘类型
textView.keyboardType = UIKeyboardType.Default
键盘的类型也是比较多的
public enum UIKeyboardType : Int {
case `default` // Default type for the current input method.
case asciiCapable // Displays a keyboard which can enter ASCII characters
case numbersAndPunctuation // Numbers and assorted punctuation.
case URL // A type optimized for URL entry (shows . / .com prominently).
case numberPad // A number pad with locale-appropriate digits (0-9, ۰-۹, ०-९, etc.). Suitable for PIN entry.
case phonePad // A phone pad (1-9, *, 0, #, with letters under the numbers).
case namePhonePad // A type optimized for entering a person's name or phone number.
case emailAddress // A type optimized for multiple email address entry (shows space @ . prominently).
@available(iOS 4.1, *)
case decimalPad // A number pad with a decimal point.
@available(iOS 5.0, *)
case twitter // A type optimized for twitter text entry (easy access to @ #)
@available(iOS 7.0, *)
case webSearch // A default keyboard type with URL-oriented addition (shows space . prominently).
@available(iOS 10.0, *)
case asciiCapableNumberPad // A number pad (0-9) that will always be ASCII digits.
public static var alphabet: UIKeyboardType { get } // Deprecated
}
自定义选择内容后的菜单
在点选文字后会弹出菜单进行选择,复制等操作。我们可以在这个菜单上添加一些其他内容,如加上“邮件”“微信”等按钮选项
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let textview = UITextView(frame:CGRect(x:10, y:100, width:200, height:100))
textview.layer.borderWidth = 1 //边框粗细
textview.layer.borderColor = UIColor.gray.cgColor //边框颜色
self.view.addSubview(textview)
let mail = UIMenuItem(title: "邮件", action: #selector(ViewController.onMail))
let weixin = UIMenuItem(title: "微信", action: #selector(ViewController.onWeiXin))
let menu = UIMenuController()
menu.menuItems = [mail,weixin]
}
@objc func onMail() {
print("mail")
}
@objc func onWeiXin() {
print("weixin")
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/25/swift-ui-multi-line-text-input-box-uitextview/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

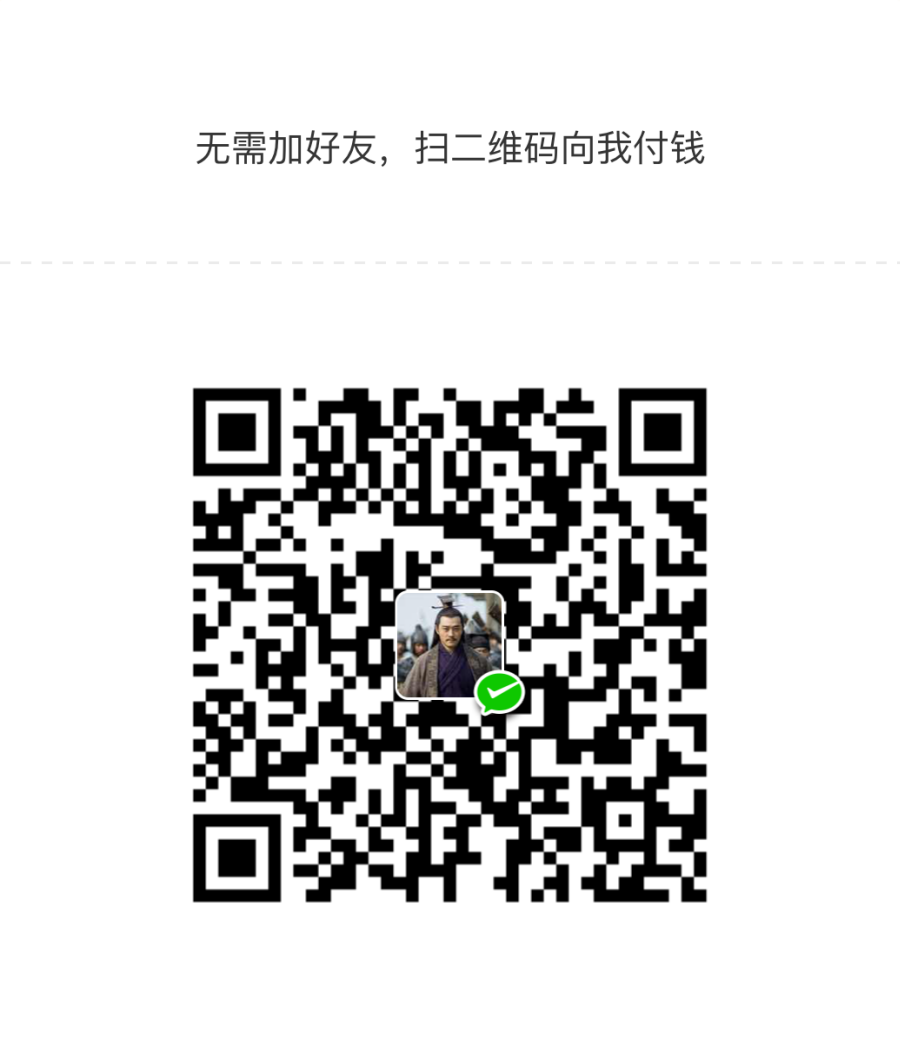
Swift UI – 多行文本输入框(UITextView)
注:代码已升级至Swift4
多行文本控件的创建
文本输入框支持的样式:
let textview = UITextView(frame:CGRect(x:10, y:100, width:200, height:100))
text……

文章目录
关闭
共有 0 条评论