TestNG编写测试
编写TestNG测试基本上包括以下步骤:
- 测试代码添加TestNG的注解
- 添加testng.xml文件
- 运行TestNG
TestNG测试流程
通过一个完整的例子,实现TestNG测试流程
POJO类:Employee.java
package me.yezhou;
public class Employee {
private String name;
private double monthlySalary;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getMonthlySalary() {
return monthlySalary;
}
public void setMonthlySalary(double monthlySalary) {
this.monthlySalary = monthlySalary;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
业务类:EmployeeBusiness.java
package me.yezhou;
public class EmployeeBusiness {
// Calculate the yearly salary of employee
public double calculateYearlySalary(Employee employee) {
double yearlySalary = 0;
yearlySalary = employee.getMonthlySalary() * 12;
return yearlySalary;
}
// Calculate the appraisal amount of employee
public double calculateAppraisal(Employee employee) {
double appraisal = 0;
if (employee.getMonthlySalary() < 10000) {
appraisal = 500;
} else {
appraisal = 1000;
}
return appraisal;
}
}
创建TestNG测试类:EmployeeTest.java
package me.yezhou;
import org.testng.Assert;
import org.testng.annotations.Test;
public class EmployeeTest {
Employee employee = new Employee();
EmployeeBusiness employeeBusiness = new EmployeeBusiness();
// test to check appraisal amount
@Test
public void testCalculateAppriasal() {
employee.setName("Joe.Ye");
employee.setAge(25);
employee.setMonthlySalary(8000);
double appraisal = employeeBusiness.calculateAppraisal(employee);
Assert.assertEquals(500.0, appraisal);
}
// test to check yearly salary
@Test
public void testCalculateYearlySalary() {
employee.setName("Joe.Ye");
employee.setAge(25);
employee.setMonthlySalary(8000);
double salary = employeeBusiness.calculateYearlySalary(employee);
Assert.assertEquals(96000.0, salary);
}
}
创建TestNG测试配置文件:testng.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="Suite">
<test name="test">
<classes>
<class name="me.yezhou.EmployeeTest"/>
</classes>
</test>
</suite>
-
标签代表一个测试套件,可以包含一个或多个测试 -
标签代表一个测试,可以包含一个或多个TestNG测试类 -
标签代表一个TestNG类是一个Java类,包含至少一个TestNG的注解,可以包含一个或多个测试方法
运行测试
IntelliJ IDEA 运行:Run D:/AutoTest/AutoTest/employee.xml
命令行运行:javac & java
# javac Employee.java EmployeeBusiness.java EmployeeTest.java
# java -cp "C:\TestNG_WORKSPACE" org.testng.TestNG testng.xml
测试报告
TestNG测试完成会在test-output目录下自动生成测试报告。使用浏览器打开index.html,页面如下:
注意:IntelliJ IDEA自动生成testNG的测试报告需要在运行配置下拉展开选择Edit Configuration,找到Listeners勾选use default reporters即可
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/02/28/testng-write-test/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

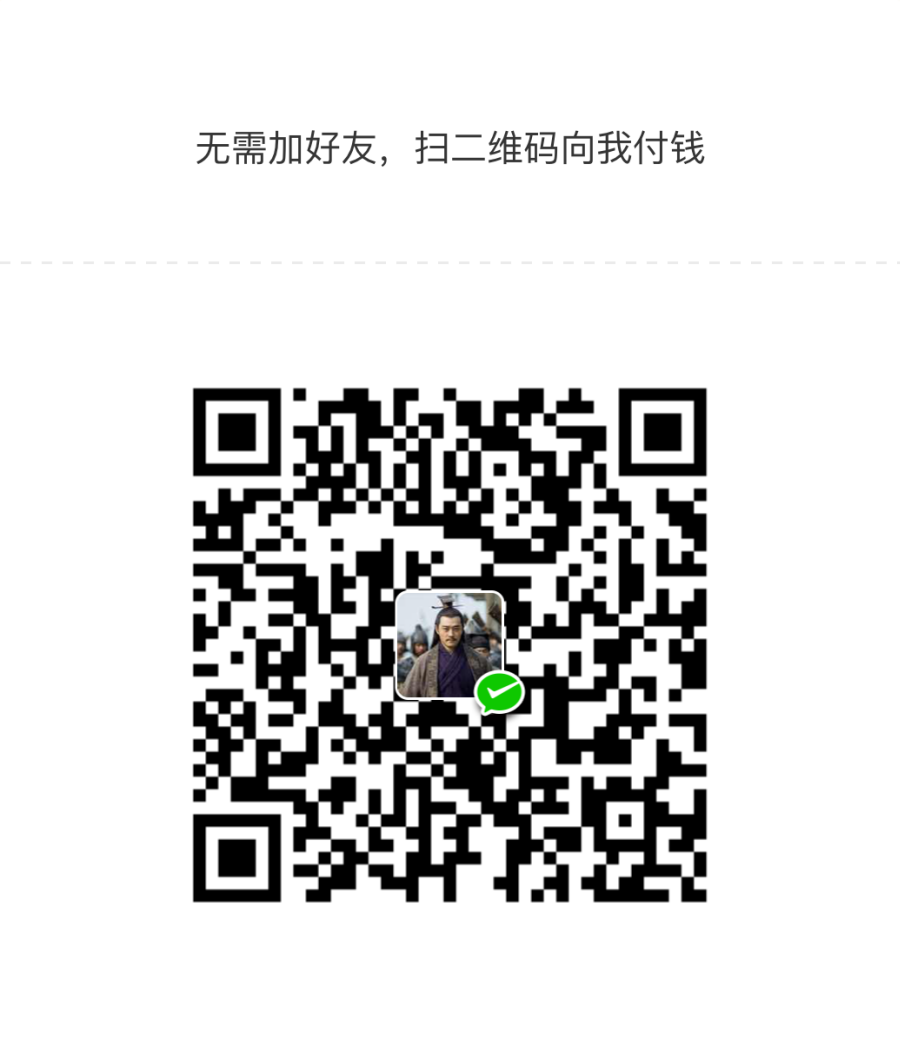

共有 0 条评论