Android ObjectAnimator记录
ObjectAnimator继承自ValueAnimator,所以ValueAnimator所能使用的方法,ObjectAnimator都可以使用,ObjectAnimator同时也重写了几个方法,比如:ofInt()
、ofFloat()
等。
基本使用
public static ObjectAnimator ofFloat(Object target, String propertyName, float... values)
- target:指定执行动画的控件
- propertyName:指定控件的属性
- values:可变长参数
//透明度动画
ObjectAnimator animator = ObjectAnimator.ofFloat(view, "alpha", 1, 0, 1);
animator.setDuration(2000);
animator.start();
//旋转动画:围绕x轴旋转
ObjectAnimator animator = ObjectAnimator.ofFloat(tv, "rotationX", 0, 270, 0);
animator.setDuration(2000);
animator.start();
//旋转动画:围绕y轴旋转
ObjectAnimator animator = ObjectAnimator.ofFloat(tv, "rotationY", 0, 180, 0);
animator.setDuration(2000);
animator.start();
//旋转动画:围绕z轴旋转
ObjectAnimator animator = ObjectAnimator.ofFloat(tv, "rotation", 0, 270, 0);
animator.setDuration(2000);
animator.start();
//平移动画:在x轴上平移
ObjectAnimator animator = ObjectAnimator.ofFloat(tv, "translationX", 0, 200, -200, 0);
animator.setDuration(2000);
animator.start();
//平移动画:在y轴上平移
ObjectAnimator animator = ObjectAnimator.ofFloat(tv, "translationY", 0, 200, -100, 0);
animator.setDuration(2000);
animator.start();
//缩放动画:在x轴上缩放
ObjectAnimator animator = ObjectAnimator.ofFloat(tv, "scaleX", 0, 3, 1);
animator.setDuration(2000);
animator.start();
//缩放动画:在y轴上缩放
ObjectAnimator animator = ObjectAnimator.ofFloat(tv, "scaleY", 0, 3, 1);
animator.setDuration(2000);
animator.start();
ObjectAnimator动画原理
ObjectAnimator动画原理:根据属性值拼装成对应的set函数的名字,比如scaleX
的拼接方法就是将属性的第一个字母强制大写后,与set拼接,也就是setScaleX
,然后通过反射找到对应控件的setScaleX(float scaleX)
函数,将当前数字值作为setScaleX(float scale)的参数将其传入。属性值的首字母大小写都可以,最终都会被强转成大写。View中都已经实现了
alpha、
rotation、
translate、
scale`相关的set方法。
自定义ObjectAnimator属性
ObjectAnimator objectAnimator = ObjectAnimator.ofInt(mCircleView,"pointRadius", 0, 200, 100, 200, 50);
objectAnimator.setDuration(1000);
objectAnimator.start();
自定义Point
public class Ponit {
private int radius;
public Ponit() {
}
public Ponit(int radius) {
this.radius = radius;
}
public int getRadius() {
return radius;
}
public void setRadius(int radius) {
this.radius = radius;
}
}
自定义view
public class CircleView extends View {
private Ponit mPoint = new Ponit();
private Paint mPiant;
private int mScreenWidth; //屏幕宽度
public CircleView(Context context) {
this(context, null);
}
public CircleView(Context context, @Nullable AttributeSet attrs) {
this(context, attrs, 0);
}
public CircleView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
mPiant = new Paint(Paint.ANTI_ALIAS_FLAG);
mPiant.setColor(Color.RED);
mPiant.setStyle(Paint.Style.FILL);
mScreenWidth = ((WindowManager)getContext().getSystemService(Context.WINDOW_SERVICE)).getDefaultDisplay().getWidth();
}
@Override
protected void onDraw(Canvas canvas) {
if (mPoint != null) {
canvas.drawCircle(mScreenWidth/2, getY()+getPaddingTop(), mPoint.getRadius(), mPiant);
}
}
public int getPointRadius() {
return mPoint.getRadius();
}
public void setPointRadius(int radius) { //set方法必须和ObjectAnimator中的属性值对应
mPoint.setRadius(radius);
invalidate();
}
}
ObjectAnimator改变控件背景颜色
以TextView为类,改变其背景颜色,关键方法是继承View的控件都实现的这个方法
public void setBackgroundColor(int color);
ObjectAnimator objectAnimator = ObjectAnimator.ofInt(tv, "backgroundColor", 0xfff10f0f, 0xff0f94f1, 0xffeaf804, 0xfff92a0f);
objectAnimator.setDuration(2000);
objectAnimator.setEvaluator(new ArgbEvaluator());
objectAnimator.start();
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/11/android-objectanimator-record/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

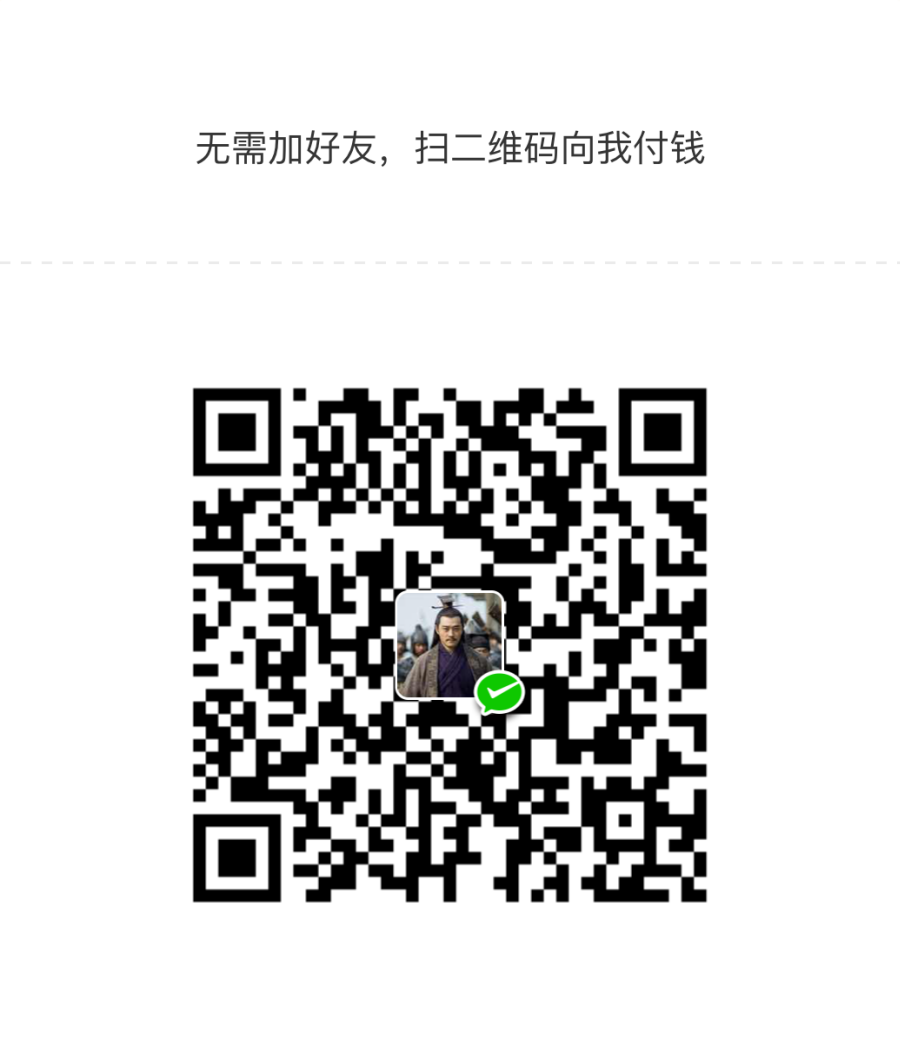

共有 0 条评论