Android Intent和PendingIntent的区别
Intent和PendingIntent的区别
- (1). Intent是立即使用的,而PendingIntent可以等到事件发生后触发,PendingIntent可以cancel
- (2). Intent在程序结束后即终止,而PendingIntent在程序结束后依然有效
- (3). PendingIntent自带Context,而Intent需要在某个Context内运行
- (4). Intent在原task中运行,PendingIntent在新的task中运行
PendingIntent
PendingIntent字面意义:等待的,未决定的Intent。
要得到一个PendingIntent对象,使用方法类的静态方法
//从系统取得一个用于启动一个Activity的PendingIntent对象
PendingIntent.getActivity(Context, int, Intent, int);
//从系统取得一个用于启动一个Service的PendingIntent对象
PendingIntent.getBroadcast(Context, int, Intent, int);
//从系统取得一个用于向BroadcastReceiver的Intent广播的PendingIntent对象
PendingIntent.getService(Context, int, Intent, int);
分别对应着Intent的3个行为,跳转到一个activity组件、打开一个广播组件和打开一个服务组件。
Intent英文意思是意图,Pending表示即将发生或来临的事情。
PendingIntent这个类用于处理即将发生的事情。比如在通知Notification中用于跳转页面,但不是马上跳转。
PendingIntent是一种特殊的Intent。主要的区别在于Intent的执行立刻的,而PendingIntent的执行不是立刻的。PendingIntent执行的操作实质上是参数传进来的Intent的操作,但是使用PendingIntent的目的在于它所包含的Intent的操作的执行是需要满足某些条件的。
主要的使用的例子:通知Notificatio的发送,短消息SmsManager的发送和警报器AlarmManager的执行等等。
Intent
Intent 是及时启动,Intent 随所在的 Activity 消失而消失。
PendingIntent 可以看作是对 Intent 的包装,由于PendingIntent中 保存有当前App的Context,使它赋予外部App一种能力,使得外部App可以如同当前App一样的执行PendingIntent里的 Intent, 就算在执行时当前App已经不存在了,也能通过存在PendingIntent里的Context照样执行Intent。另外还可以处理Intent执行后的操作。常和AlarmManager 和NotificationManager一起使用。
Intent一般是用作Activity、Sercvice、BroadcastReceiver之间传递数据,而Pendingintent,一般用在 Notification上,可以理解为延迟执行的intent,PendingIntent是对Intent一个包装。
Android的状态栏通知(Notification)
如果需要查看消息,可以拖动状态栏到屏幕下方即可查看消息。步骤:
- (1)获取通知管理器NotificationManager,它也是一个系统服务
- (2)建立通知Notification notification = new Notification(icon, null, when);
- (3)为新通知设置参数(比如声音,震动,灯光闪烁)
- (4)把新通知添加到通知管理器
发送消息的代码如下:
//获取通知管理器
NotificationManager mNotificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE)
int icon = android.R.drawable.stat_notify_chat;
long when = System.currentTimeMillis(); //通知发生的时间为系统当前时间
//新建一个通知,指定其图标和标题
Notification notification = new Notification(icon, null, when); //第一个参数为图标,第二个参数为短暂提示标题,第三个为通知时间
notification.defaults = Notification.DEFAULT_SOUND; //发出默认声音
notification.flags |= Notification.FLAG_AUTO_CANCEL; //点击通知后自动清除通知
Intent openintent = new Intent(this, OtherActivity.class);
PendingIntent contentIntent = PendingIntent.getActivity(this, 0, openintent, 0); //当点击消息时就会向系统发送openintent意图
notification.setLatestEventInfo(this, "标题", "我是内容", contentIntent);
mNotificationManager.notify(0, notification); //第一个参数为自定义的通知唯一标识
重点是setLatestEventInfo方法的PendingIntent参数,PendingIntent主要持有的信息是它所包装的Intent和当前Application的Context。正由于PendingIntent中保存有当前Application的Context,使它赋予带他程序一种执行的Intent的能力,就算在执行时当前Application已经不存在了,也能通过存在PendingIntent里的Context照样执行Intent。
private void showNotify() {
Notification notice = new Notification();
notice.icon = R.drawable.icon;
notice.tickerText = "您有一条新的信息";
notice.defaults = Notification.DEFAULT_SOUND;
notice.when = 10L;
// 100 毫秒延迟后,震动 250 毫秒,暂停 100 毫秒后,再震动 500 毫秒
//notice.vibrate = new long[] { 100, 250, 100, 500 };
//notice.setLatestEventInfo(this, "通知", "开会啦", PendingIntent.getActivity(this, 0, null, 0));
notice.setLatestEventInfo(this, "通知", "开会啦", PendingIntent.getActivity(this, 0, new Intent(this, Activity2.class), 0)); //即将跳转页面,还没跳转
NotificationManager manager = (NotificationManager)getSystemService(this.NOTIFICATION_SERVICE);
manager.notify(0, notice);
}
SmsManager发送短信
SmsManager的用于发送短信的方法:
SmsManager.sendTextMessage(String destinationAddress, String scAddress, String text, PendingIntent sentIntent, PendingIntent deliveryIntent)
- 第一个参数:destinationAddress对方手机号码
- 第二个参数:scAddress短信中心号码一般设置为空
- 第三个参数:text短信内容
- 第四个参数:sentIntent判断短信是否发送成功,当短信发出时,成功的话sendIntent会把其内部的描述的Intent广播出去,否则产生错误代码并通过android.app.PendingIntent.OnFinished进行回调,这个参数最好不为空,否则会存在资源浪费的潜在问题;
- 第五个参数:deliveryIntent当短信发送到收件人时,会收到这个deliveryIntent。即强调了“发送”后的结果
就是说是在"短信发送成功"和"对方收到此短信"才会激活 sentIntent和deliveryIntent这两个Intent。这也相当于是延迟执行了Intent。
Android发送短信示例:
String msg = "你好,美女";
String number = "135****6784";
SmsManager sms = SmsManager.getDefault();
//当短信发送成功后进行的PendingIntent广播
//当消息已经传递给收信人后所进行的PendingIntent广播
PendingIntent pi = PendingIntent.getBroadcast(SmsActivity.this, 0, new Intent(...), 0);
sms.sendTextMessage(number, null, msg, pi, null);
Toast.makeText(SmsActivity.this, "发送成功", Toast.LENGHT_LONG).show();
PendingIntent就是一个Intent的描述,我们可以把这个描述交给别的程序,别的程序根据这个描述在后面的别的时间做你安排做的事情 。本例中别的程序就是发送短信的程序,短信发送成功后要把Intent广播出去。
上面两个例子可以理解,PendingIntent就是一个可以在满足一定条件下执行的Intent,它相比于Intent的优势在于自己携带有Context对象,这样他就不必依赖于某个activity才可以存在。
总结
PendingIntent根据字面意思就知道是延迟的intent,主要用来在某个事件完成后执行特定的Action。PendingIntent包含了Intent及Context,所以就算Intent所属程序结束,PendingIntent依然有效,可以在其他程序中使用。
PendingIntent常用在通知栏及短信发送系统中。PendingIntent一般作为参数传给某个实例,在该实例完成某个操作后自动执行PendingIntent上的Action,也可以通过PendingIntent的send函数手动执行,并可以在send函数中设置OnFinished表示send成功后执行的动作。
即使PendingIntent原进程结束,PendingIntent本身仍然存在,可在其他进程(PendingIntent被递交到的其他程序)中继续使用。
当你把PendingIntent递交给别的程序进行处理时,PendingIntent仍然拥有PendingIntent原程序所拥有的权限(with the same permissions and identity)。
短信系统举例代码
private final static String SEND_ACTION = "send";
private final static String DELIVERED_ACTION = "delivered";
private void sendSms(String receiver, String text) {
SmsManager s = SmsManager.getDefault();
PendingIntent sentPI = PendingIntent.getBroadcast(this, 0, new Intent(SEND_ACTION), PendingIntent.FLAG_CANCEL_CURRENT);
PendingIntent deliveredPI = PendingIntent.getBroadcast(this, 0, new Intent(DELIVERED_ACTION), PendingIntent.FLAG_CANCEL_CURRENT);
// 发送完成
registerReceiver(new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
switch (getResultCode()) {
case Activity.RESULT_OK:
Toast.makeText(getBaseContext(), "Send Success!", Toast.LENGTH_SHORT).show();
break;
case SmsManager.RESULT_ERROR_GENERIC_FAILURE:
Toast.makeText(getBaseContext(), "Send Failed because generic failure cause.", Toast.LENGTH_SHORT).show();
break;
case SmsManager.RESULT_ERROR_NO_SERVICE:
Toast.makeText(getBaseContext(), "Send Failed because service is currently unavailable.", Toast.LENGTH_SHORT).show();
break;
case SmsManager.RESULT_ERROR_NULL_PDU:
Toast.makeText(getBaseContext(), "Send Failed because no pdu provided.", Toast.LENGTH_SHORT).show();
break;
case SmsManager.RESULT_ERROR_RADIO_OFF:
Toast.makeText(getBaseContext(), "Send Failed because radio was explicitly turned off.", Toast.LENGTH_SHORT).show();
break;
default:
Toast.makeText(getBaseContext(), "Send Failed.", Toast.LENGTH_SHORT).show();
break;
}
}
}, new IntentFilter(SEND_ACTION));
// 对方接受完成
registerReceiver(new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
switch (getResultCode()) {
case Activity.RESULT_OK:
Toast.makeText(getBaseContext(), "Delivered Success!", Toast.LENGTH_SHORT).show();
break;
default:
Toast.makeText(getBaseContext(), "Delivered Failed!", Toast.LENGTH_SHORT).show();
break;
}
}
}, new IntentFilter(DELIVERED_ACTION));
// 发送短信,sentPI和deliveredPI将分别在短信发送成功和对方接受成功时被广播
s.sendTextMessage(receiver, null, text, sentPI, deliveredPI);
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/11/difference-between-android-intent-and-pendingintent/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

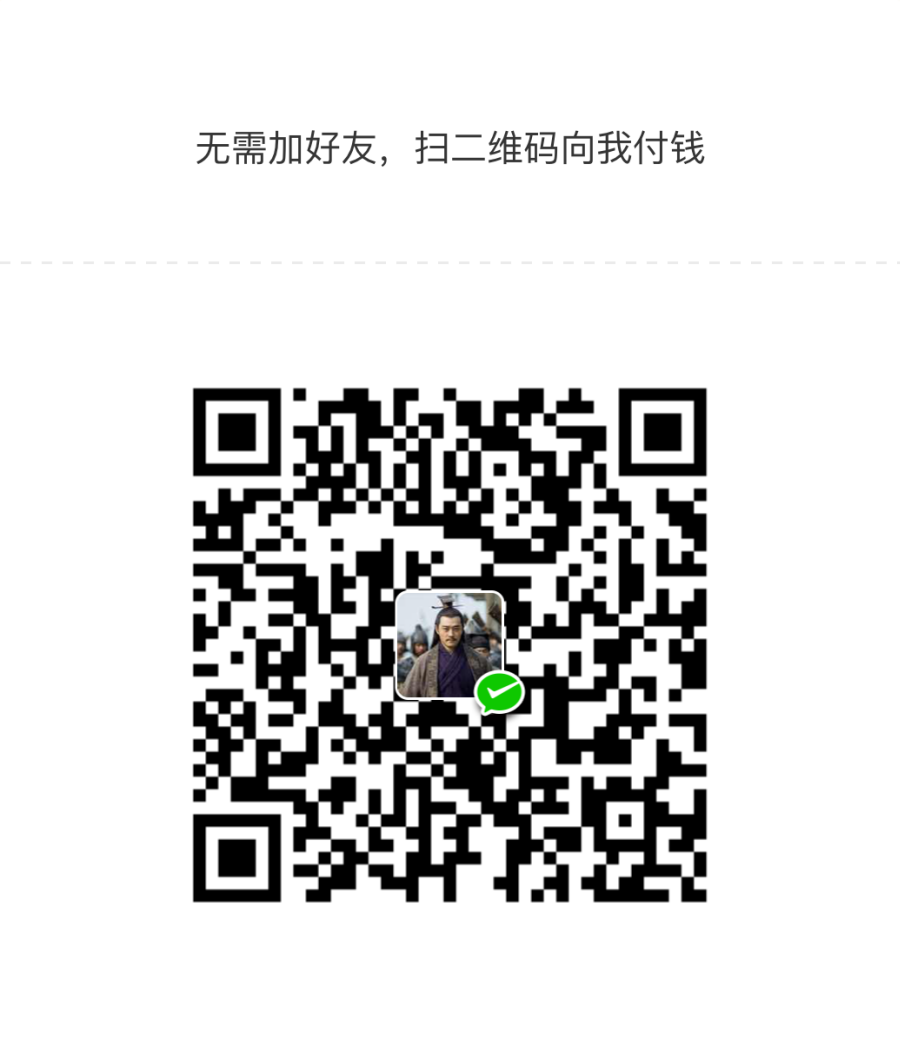

共有 0 条评论