Flutter中Json解析
json.decode
json.decode返回值为Map<String, dynamic>直到运行的时候才能知道具体的类型
所以不能:类型检查,自动提示,编译时无法发现错误
json_serializable
(1)pubspec.yaml中添加依赖
dependencies:
json_annotation: ^2.0.0 # https://pub.dartlang.org/packages/json_annotation
dev_dependencies:
build_runner: ^1.1.2 # https://pub.dartlang.org/packages/build_runner
json_serializable: ^2.0.1 # https://pub.dartlang.org/packages/json_serializable
(2)创建Model并添加注解
import 'package:json_annotation/json_annotation.dart';
part 'person.g.dart';
@JsonSerializable()
class Person {
String name;
int age;
Person(this.name, this.age);
factory Person.fromJson(Map<String, dynamic> json) => _$PersonFromJson(json);
Map<String, dynamic> toJson() => _$PersonToJson(this);
}
(3)自动生成fromJson和toJson(model.g.dart)
flutter packages pub run build_runner build
代码示例
import 'package:flutter/material.dart';
import 'dart:convert';
import 'package:flutter_app/model/person.dart';
class JsonDemoPage extends StatefulWidget {
@override
State<StatefulWidget> createState() => new _JsonDemoPagePageState();
}
class _JsonDemoPagePageState extends State<JsonDemoPage> {
String name = "test";
User user = null;
Person person = null;
@override
void initState() {
super.initState();
}
@override
void didUpdateWidget(covariant JsonDemoPage oldWidget) {
name = JsonDecoder.parseJson2Map();
user = JsonDecoder.parseJson2Object();
person = JsonDecoder.parseJsonSerialize();
}
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(
title: new Text('JSON Demo'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('name: $name'),
SizedBox(height: 10,),
Text('User: [name: ${user.name}, homepage: ${user.homepage}]', textAlign: TextAlign.center,),
SizedBox(height: 10,),
Text('Person: ${person.toJson()}', textAlign: TextAlign.center,),
],
)
),
);
}
}
class JsonDecoder {
//json.decode返回值为Map<String, dynamic>直到运行的时候才能知道具体的类型
//所以不能:类型检查,自动提示,编译时无法发现错误,严重不推荐!
static String parseJson2Map() {
String jsonStr = '{"name": "Joe.Ye"}';
var user = json.decode(jsonStr);
print('${user['name']}');
return '${user['name']}';
}
//手写fromJson, toJson不太友好
static User parseJson2Object() {
String jsonStr = '{"name":"Joe.Ye", "email": "yezhou@yezhou.org", "homepage":"http://www.appblog.cn"}';
Map userMap = json.decode(jsonStr);
var user = new User.fromJson(userMap);
print('${user.name}');
print(user.toJson());
return user;
}
static Person parseJsonSerialize() {
String jsonStr = '{"name":"Joe.Ye", "age":30}';
Map personMap = json.decode(jsonStr);
return Person.fromJson(personMap);
}
//https://javiercbk.github.io/json_to_dart/
}
class User {
final String name;
final String email;
final String homepage;
User(this.name, this.email, this.homepage);
User.fromJson(Map<String, dynamic> json)
: name = json['name'],
email = json['email'],
homepage = json['homepage'];
Map<String, dynamic> toJson() => {
'name': name,
'email': email,
'homepage': homepage
};
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/16/flutter-json-parsing/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

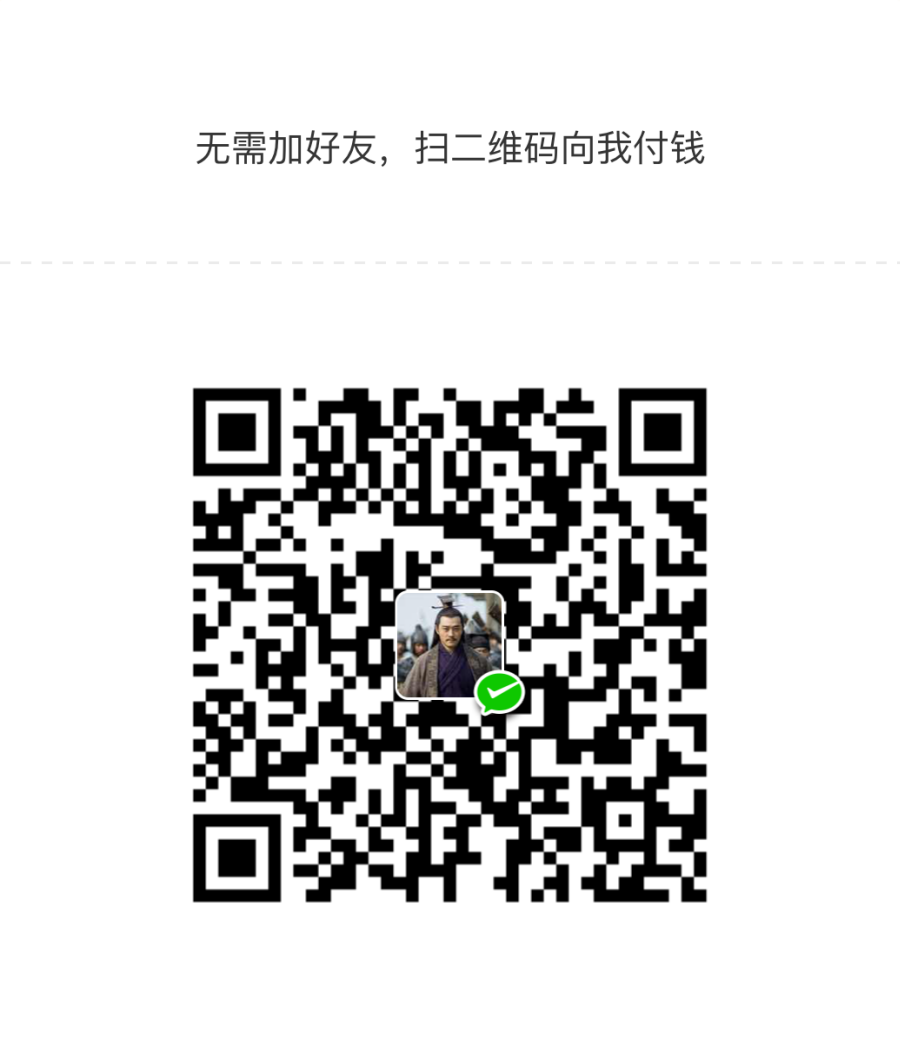
Flutter中Json解析
json.decode
json.decode返回值为Map<String, dynamic>直到运行的时候才能知道具体的类型
所以不能:类型检查,自动提示,编译时无法发现错误
json_ser……

文章目录
关闭
共有 0 条评论