通过javax.validation.constraints下的注解实现字段验证
添加maven依赖包
<!-- https://mvnrepository.com/artifact/javax.validation/validation-api -->
<dependency>
<groupId>javax.validation</groupId>
<artifactId>validation-api</artifactId>
</dependency>
在校验字段上添加校验注解
class Person {
@NotNull(message = "字段值不能为空")
private String name;
@NotNull
private String sex;
@Max(value = 20, message = "最大长度为20")
private String address;
@NotNull
@Size(max = 10, min = 5, message = "字段长度要在5-10之间")
private String fileName;
@Pattern(regexp = "^[a-zA-Z0-9_.-]+@[a-zA-Z0-9-]+(\\.[a-zA-Z0-9-]+)*\\.[a-zA-Z0-9]{2,6}$", message = "不满足邮箱正则表达式")
private String email;
@AssertTrue(message = "字段为true才能通过")
private boolean isSave;
@Future(message = "时间在当前时间之后才可以通过")
private Date date;
}
在控制器接收参数时,添加@Valid
注解,例如:
public String index(@Valid Person person, BindingResult result)
可以通过BindingResult对象获取相关的错误提示。
也可以自定义验证方式
@Component
public class ValidatorHelper {
@Autowired
private Validator validator;
public <T> Pair<Boolean, String> validate(T request) {
if (request == null) {
return Pair.of(Boolean.FALSE, "request is null");
}
Set<ConstraintViolation<T>> r = validator.validate(request);
Iterator<ConstraintViolation<T>> it = r.iterator();
if (it.hasNext()) {
ConstraintViolation<T> c = it.next();
return Pair.of(Boolean.FALSE, String.format("[%s]%s", c.getPropertyPath(), c.getMessage()));
}
return Pair.of(Boolean.TRUE, "validate success");
}
}
验证的注解
(1)@NotNull
使用该注解的字段的值不能为null,否则验证无法通过
(2)@Null
修饰的字段在验证时必须是null,否则验证无法通过
(3)@Size
如下代码表示,修饰的字段长度不能超过5或者低于1
@Size(min = 1, max = 5)
private String name;
(4)@Max
如下代码表示,该字段的最大值为19,否则无法通过验证
@Max(value = 19)
private Integer age;
(5)@Min
同理,被该注解修饰的字段的最小值,不能低于某个值
(6)@AssertFalse
该字段值为false时,验证才能通过
(7)`@AssertTrue
该字段值为true时,验证才能通过
(8)@DecimalMax
验证小数的最大值
@DecimalMax(value = "12.35")
private double money;
(9)@DecimalMin
验证小数的最小值
(10)@Digits
验证数字的整数位和小数位的位数是否超过指定的长度
@Digits(integer = 2, fraction = 2)
private double money;
(11)@Future
验证日期是否在当前时间之后,否则无法通过校验
@Future
private Date date;
(12)@Past
验证日期是否在当前时间之前,否则无法通过校验
(13)@Pattern
用于验证字段是否与给定的正则相匹配
@Pattern(regexp = "[abc]")
private String name;
@NotEmpty, @NotNull, @NotBlank 的区别
(1)@NotEmpty
The annotated element must not be {@code null} nor empty.
不能是null
不能是空字符
集合框架中的元素不能为空
(2)@NotNul
被修饰元素不能为null,可用在基本类型上
(3)@NotBlank
The annotated element must not be {@code null} and must contain at least one non-whitespace character.
该注解用来判断字符串或者字符,只用在String上面
如果在基本类型上面用@NotEmpty
或者@NotBlank
,会出现以下错误
org.springframework.web.util.NestedServletException: Request processing failed; nested exception is javax.validation.UnexpectedTypeException: HV000030: No validator could be found for constraint 'javax.validation.constraints.NotBlank' validating type 'java.lang.Long'. Check configuration for 'offset'
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/19/implement-field-validation-through-annotations-under-javax-validation-constraints/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

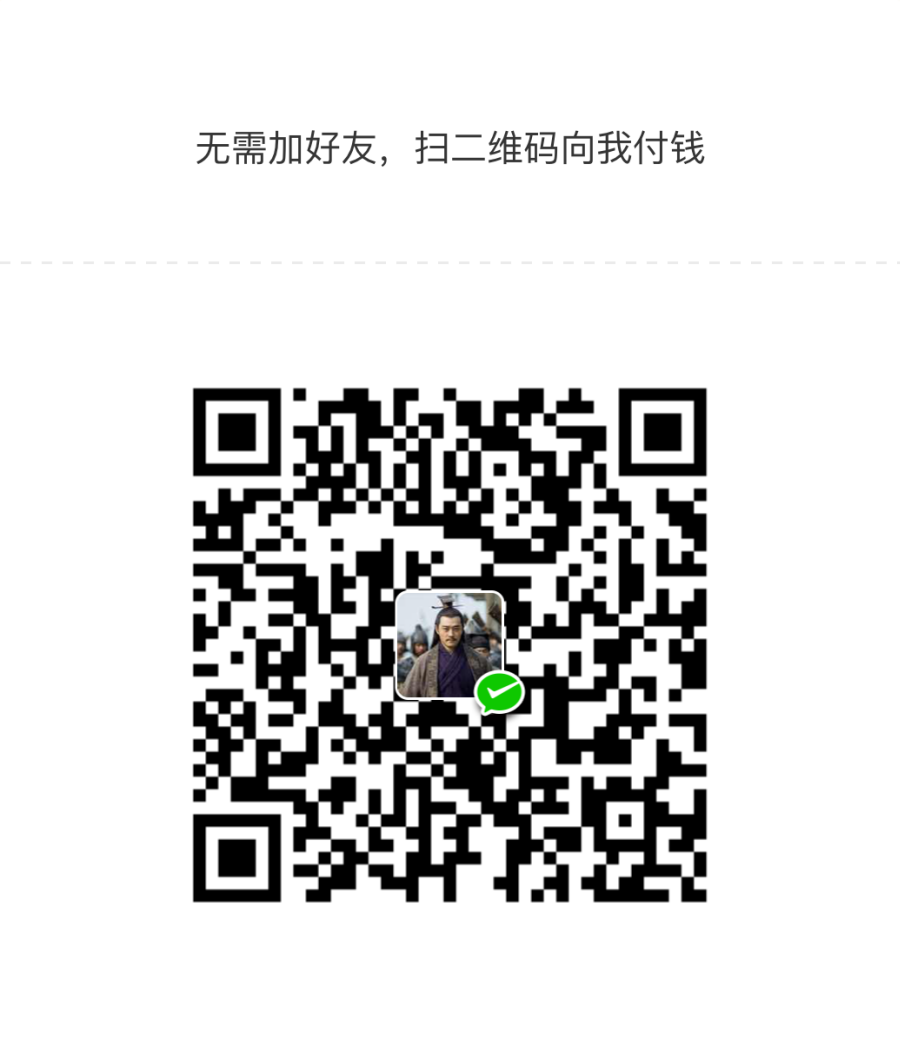

共有 0 条评论