Spring Security OAuth2 Redis 资源服务器配置
资源服务器相关依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
<version>2.3.6.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
资源服务器配置类
/**
* @Description: @EnableResourceServer注解实际上相当于加上OAuth2AuthenticationProcessingFilter过滤器
* @Package: cn.appblog.security.oauth2.config.ResServerConfig
* @Version: 1.0
*/
@Configuration
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Autowired
private RedisConnectionFactory redisConnectionFactory;
@Autowired
private UserAuthenticationEntryPoint userAuthenticationEntryPoint;
@Autowired
private UserAccessDeniedHandler userAccessDeniedHandler;
@Autowired
private UserAuthenticationSuccessHandler userAuthenticationSuccessHandler;
@Override
public void configure(ResourceServerSecurityConfigurer resources) throws Exception {
resources
.tokenServices(tokenServices())
//资源ID
.resourceId("resource_password_id")
//用来解决匿名用户访问无权限资源时的异常
.authenticationEntryPoint(userAuthenticationEntryPoint)
//访问资源权限相关异常处理
.accessDeniedHandler(userAccessDeniedHandler);
}
@Override
public void configure(HttpSecurity http) throws Exception {
http.csrf().disable();
http
.authorizeRequests()
.antMatchers("/resource/auth")
.denyAll()
.and()
.authorizeRequests()
.anyRequest()
.permitAll();
}
/**
* OAuth2 token持久化接口
*/
@Bean
public TokenStore tokenStore() {
return new RedisTokenStore(redisConnectionFactory);
}
/**
* 令牌服务
*/
@Bean
public DefaultTokenServices tokenServices() {
DefaultTokenServices defaultTokenServices = new DefaultTokenServices();
defaultTokenServices.setTokenStore(tokenStore());
return defaultTokenServices;
}
/**
* 加密方式
*/
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
配置文件
server.port=9004
##单机应用环境配置
spring.redis.host=127.0.0.1
spring.redis.port=6379
#spring.redis.password=
spring.redis.database=0
#spring.redis.timeout=
##redis连接池配置
## 连接池中的最小空闲连接,默认0
spring.redis.jedis.pool.min-idle=0
## 连接池中的最大空闲连接,默认8
spring.redis.jedis.pool.max-idle=8
## 连接池最大阻塞等待时间(使用负值表示没有限制),默认-1ms
spring.redis.jedis.pool.max-wait=-1ms
##连接池最大连接数(使用负值表示没有限制),默认8
spring.redis.jedis.pool.max-active=8
oauth.token.uri=http://127.0.0.1:9003/oauth/token
oauth.resource.id=resource_password_id
oauth.resource.client.id=client_password
oauth.resource.client.secret=secret
oauth.resource.user.id=user
oauth.resource.user.password=123456
资源控制器
/**
* @Description: 资源服务器
* @Package: cn.appblog.security.oauth2.api.ResourceController
* @Version: 1.0
*/
@Slf4j
@RestController
@RequestMapping("/resource")
public class ResourceController {
@RequestMapping(value = "context", method = RequestMethod.GET)
public ResponseEntity<Object> get(@RequestParam String username, @RequestParam int age) {
SecurityContext ctx = SecurityContextHolder.getContext();
log.info(JSON.toJSONString(ctx));
if (!"anonymousUser".equals(ctx.getAuthentication().getPrincipal())) {
return new ResponseEntity<>(ctx, HttpStatus.OK);
}
return new ResponseEntity<>(ctx, HttpStatus.OK);
}
@RequestMapping(value = "auth", method = RequestMethod.GET)
@ResponseBody
public Object getAuth() {
SecurityContext ctx = SecurityContextHolder.getContext();
return ctx.getAuthentication();
}
}
访问测试
(1)访问:http://127.0.0.1:9004/resource/context?username=joe&age=20
{
"authentication":{
"authorities":[
{
"authority":"ROLE_ANONYMOUS"
}
],
"details":{
"remoteAddress":"127.0.0.1",
"sessionId":null
},
"authenticated":true,
"principal":"anonymousUser",
"keyHash":-872685687,
"credentials":"",
"name":"anonymousUser"
}
}
{
"authentication":{
"authenticated":true,
"authorities":[
{
"authority":"{"interfaces":["/a/b","/a/c","/oauth/token"]}"
},
{
"authority":"{"username":"user"}"
}
],
"clientOnly":false,
"credentials":"",
"details":{
"remoteAddress":"127.0.0.1",
"tokenType":"Bearer",
"tokenValue":"b970020dc0e942d0ae4a0e01232ff05f"
},
"name":"user",
"oAuth2Request":{
"approved":true,
"authorities":[
],
"clientId":"client_password",
"extensions":{
},
"grantType":"password",
"refresh":false,
"requestParameters":{
"grant_type":"password",
"client_id":"client_password",
"username":"user"
},
"resourceIds":[
"resource_password_id"
],
"responseTypes":[
],
"scope":[
"all"
]
},
"principal":{
"accountNonExpired":true,
"accountNonLocked":true,
"authorities":[
{
"$ref":"$.authentication.authorities[0]"
},
{
"$ref":"$.authentication.authorities[1]"
}
],
"credentialsNonExpired":true,
"enabled":true,
"username":"user"
},
"userAuthentication":{
"authenticated":true,
"authorities":[
{
"$ref":"$.authentication.authorities[0]"
},
{
"$ref":"$.authentication.authorities[1]"
}
],
"details":{
"client_secret":"secret",
"grant_type":"password",
"client_id":"client_password",
"username":"user"
},
"name":"user",
"principal":{
"$ref":"$.authentication.principal"
}
}
}
}
{
"status": 300,
"message": "登录异常,请检查登录信息...,org.springframework.security.authentication.InsufficientAuthenticationException: Invalid access token: b970020dc0e942d0ae4a0e01232ff05f"
}
本文转载参考 原文 并加以调试
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/19/spring-security-oauth2-redis-resource-server-configuration/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

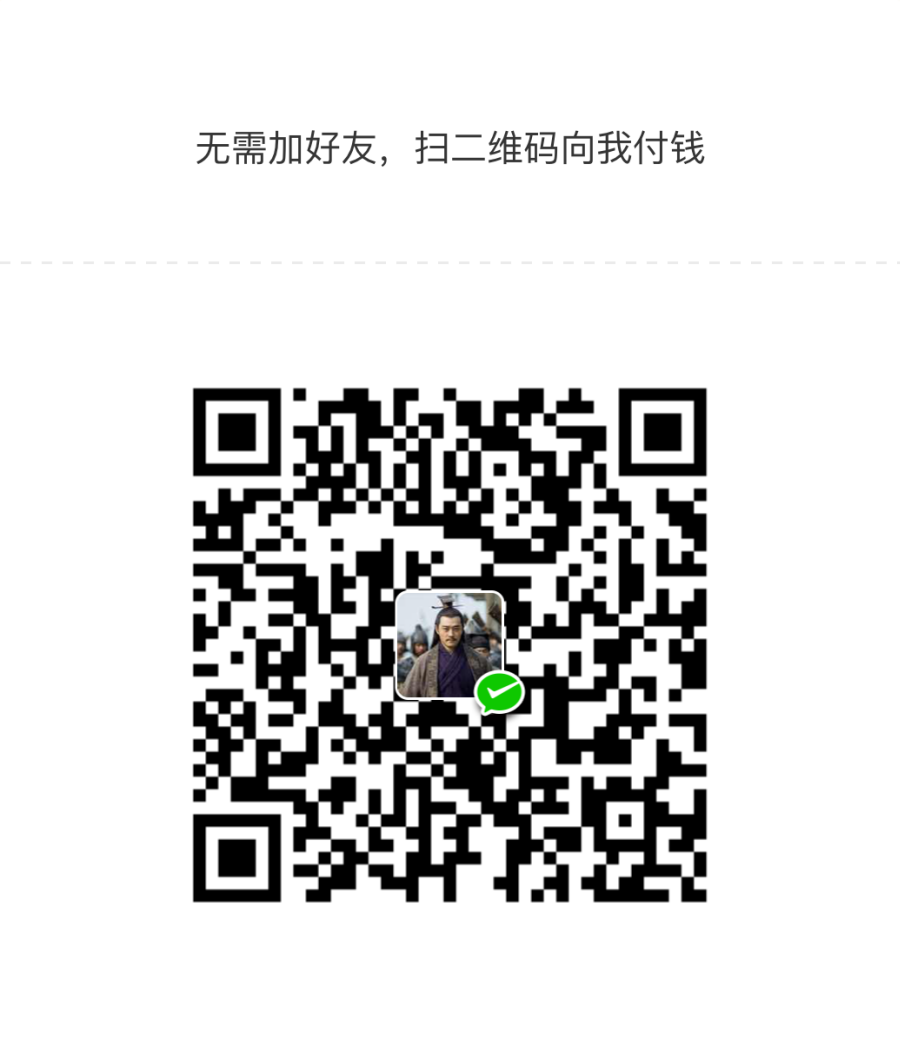
Spring Security OAuth2 Redis 资源服务器配置
资源服务器相关依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security……

文章目录
关闭
共有 0 条评论