Kubernetes api微服务开发之pod创建与删除
目标:完成Pod创建删除服务的开发
Kubernetes Client简介
kubernetes client为fabric8框架下的kubernetes api封装,提供了多种操作类,可调用API Server完成相应功能。
Github地址:https://github.com/fabric8io/kubernetes-client.git
Kubernetes Pod YAML文件说明
首先在kubernetes master上编写Pod的YAML文件,测试Pod的创建删除功能是否可用。
vim Pod-test.yaml
apiVersion: v1
kind: Pod
metadata:
name: nginx-1.17.0
namespace: appblog
spec:
containers:
- name: nginx-1170
image: nginx
ports:
- containerPort: 80
hostPort: 30011
其中kind表示需要使用的类别,metadata为基本信息,spec包含容器信息。
在Master节点上执行:
kubectl create -f Pod-test.yaml //创建pod
kubectl delete -f Pod-test.yaml //删除pod
Pod服务编写
新增pod创建与删除代码:
(1)服务类-DevK8sApiService.java
//创建Pod
public Pod createPod(String nameSpace, String podName, String containerName, String imageName, int cnPort, int htPort) {
//ObjectMeta 配置
ObjectMeta objectMeta = new ObjectMetaBuilder().
withName(podName).
withNamespace(nameSpace).
build();
//Container 端口配置
ContainerPort containerPort = new ContainerPortBuilder().
withContainerPort(cnPort).
withHostPort(htPort).
build();
//Container 配置
Container container = new ContainerBuilder().
withName(containerName).
withImage(imageName).
withPorts(containerPort).
build();
//Spec 配置
PodSpec podSpec = new PodSpecBuilder().
withContainers(container).
build();
//Pod 配置
Pod pod = new PodBuilder().
withApiVersion("v1").
withKind("Pod").
withMetadata(objectMeta).
withSpec(podSpec).
build();
try {
//Pod 创建
kubernetesClient.pods().create(pod);
log.error("pod create success");
} catch (Exception e) {
log.error("pod create failed");
log.error("", e);
}
return pod;
}
//删除pod
public Pod deletePod(String namespaceName, String podName) {
Pod pod = new Pod();
try {
//获取要删除的pod
pod = kubernetesClient.pods().inNamespace(namespaceName).withName(podName).get();
//Pod 删除
kubernetesClient.pods().inNamespace(namespaceName).withName(podName).delete();
log.error("pod delete success");
} catch (Exception e) {
log.error("pod delete failed");
log.error("", e);
}
return pod;
}
(2)控制类-DevK8sApiController.java
//k8s pod create
@RequestMapping(value = "/createPod", method = RequestMethod.POST)
public Pod createK8sPod(@RequestParam(value = "NameSpaceName") String nsName,
@RequestParam(value = "PodName") String pdName,
@RequestParam(value = "ContainerName") String ctName,
@RequestParam(value = "ImageName") String imName,
@RequestParam(value = "ContainerPort") int cnPort,
@RequestParam(value = "HostPort") int htPort){
return devK8sApiService.createPod(nsName,pdName,ctName,imName, cnPort, htPort);
}
//k8s pod delete
@RequestMapping(value = "/deletePod", method = RequestMethod.DELETE)
public Pod deleteK8sPod(@RequestParam(value = "NameSpaceName") String nsName,
@RequestParam(value = "PodName") String pdName) {
return devK8sApiService.deletePod(nsName, pdName);
}
测试验证
(1)添加pod
POST
: http://127.0.0.1:8080/k8s/createPod
Content-Type
: application/x-www-form-urlencoded
Body
: {"NameSpaceName":"appblog", "PodName":"nginx-1.17.0", "ContainerName":"nginx-1170", "ImageName":"nginx", "ContainerPort":"80", "HostPort":"30080"}
{
"apiVersion": "v1",
"kind": "Pod",
"metadata": {
"annotations": {},
"labels": {},
"name": "nginx-1.17.0",
"namespace": "appblog"
},
"spec": {
"containers": [
{
"image": "nginx",
"name": "nginx-1170",
"ports": [
{
"containerPort": 80,
"hostPort": 30080
}
]
}
],
"nodeSelector": {}
}
}
(2)kubectl get pods -n appblog
查看
[root@k8s-master ~]# kubectl get pods -n appblog
NAME READY STATUS RESTARTS AGE
nginx-1.17.0 1/1 Running 0 4m34s
[root@k8s-master ~]# kubectl describe pod nginx-1.17.0 -n appblog
Name: nginx-1.17.0
Namespace: appblog
Priority: 0
PriorityClassName: <none>
Node: k8s-node01/192.168.0.10
Start Time: Thu, 13 Jun 2019 22:19:17 +0800
Labels: <none>
Annotations: cni.projectcalico.org/podIP: 172.16.1.9/32
Status: Running
IP: 172.16.1.9
Containers:
nginx-1170:
Container ID: docker://a37264ab1de6ed7b20859370d62b39b3854449145cf347dbbb7fe78faab33128
Image: nginx
Image ID: docker-pullable://nginx@sha256:bdbf36b7f1f77ffe7bd2a32e59235dff6ecf131e3b6b5b96061c652f30685f3a
Port: 80/TCP
Host Port: 30080/TCP
State: Running
Started: Thu, 13 Jun 2019 22:20:07 +0800
Ready: True
Restart Count: 0
Environment: <none>
Mounts:
/var/run/secrets/kubernetes.io/serviceaccount from default-token-jrbkh (ro)
Conditions:
Type Status
Initialized True
Ready True
ContainersReady True
PodScheduled True
Volumes:
default-token-jrbkh:
Type: Secret (a volume populated by a Secret)
SecretName: default-token-jrbkh
Optional: false
QoS Class: BestEffort
Node-Selectors: <none>
Tolerations: node.kubernetes.io/not-ready:NoExecute for 300s
node.kubernetes.io/unreachable:NoExecute for 300s
Events:
Type Reason Age From Message
---- ------ ---- ---- -------
Normal Scheduled 4m43s default-scheduler Successfully assigned appblog/nginx-1.17.0 to k8s-node01
Normal Pulling 4m40s kubelet, k8s-node01 Pulling image "nginx"
Normal Created 3m54s kubelet, k8s-node01 Created container nginx-1170
Normal Started 3m53s kubelet, k8s-node01 Started container nginx-1170
[root@k8s-master ~]#
以NodePort方式访问服务:http://NodeIP:30080
注:NodeIP为节点
k8s-node01的IP
(3)删除pod
DELETE
: http://127.0.0.1:8080/k8s/deletePod
Content-Type
: application/x-www-form-urlencoded
Body
: {"NameSpaceName":"appblog", "PodName:nginx-1.17.0"}
{
"apiVersion": "v1",
"kind": "Pod",
"metadata": {
"annotations": {
"cni.projectcalico.org/podIP": "172.16.1.9/32"
},
"creationTimestamp": "2019-06-13T14:19:17Z",
"name": "nginx-1.17.0",
"namespace": "appblog",
"resourceVersion": "323516",
"selfLink": "/api/v1/namespaces/appblog/pods/nginx-1.17.0",
"uid": "3a84aba5-8de6-11e9-b4ff-525400a204f6"
},
"spec": {
"containers": [
{
"image": "nginx",
"imagePullPolicy": "Always",
"name": "nginx-1170",
"ports": [
{
"containerPort": 80,
"hostPort": 30080,
"protocol": "TCP"
}
],
"resources": {},
"terminationMessagePath": "/dev/termination-log",
"terminationMessagePolicy": "File",
"volumeMounts": [
{
"mountPath": "/var/run/secrets/kubernetes.io/serviceaccount",
"name": "default-token-jrbkh",
"readOnly": true
}
]
}
],
"dnsPolicy": "ClusterFirst",
"enableServiceLinks": true,
"nodeName": "k8s-node01",
"priority": 0,
"restartPolicy": "Always",
"schedulerName": "default-scheduler",
"securityContext": {},
"serviceAccount": "default",
"serviceAccountName": "default",
"terminationGracePeriodSeconds": 30,
"tolerations": [
{
"effect": "NoExecute",
"key": "node.kubernetes.io/not-ready",
"operator": "Exists",
"tolerationSeconds": 300
},
{
"effect": "NoExecute",
"key": "node.kubernetes.io/unreachable",
"operator": "Exists",
"tolerationSeconds": 300
}
],
"volumes": [
{
"name": "default-token-jrbkh",
"secret": {
"defaultMode": 420,
"secretName": "default-token-jrbkh"
}
}
]
},
"status": {
"conditions": [
{
"lastTransitionTime": "2019-06-13T14:19:17Z",
"status": "True",
"type": "Initialized"
},
{
"lastTransitionTime": "2019-06-13T14:20:08Z",
"status": "False",
"type": "Ready"
},
{
"lastTransitionTime": "2019-06-13T14:20:08Z",
"status": "True",
"type": "ContainersReady"
},
{
"lastTransitionTime": "2019-06-13T14:19:17Z",
"status": "True",
"type": "PodScheduled"
}
],
"containerStatuses": [
{
"containerID": "docker://a37264ab1de6ed7b20859370d62b39b3854449145cf347dbbb7fe78faab33128",
"image": "nginx:latest",
"imageID": "docker-pullable://nginx@sha256:bdbf36b7f1f77ffe7bd2a32e59235dff6ecf131e3b6b5b96061c652f30685f3a",
"lastState": {},
"name": "nginx-1170",
"ready": true,
"restartCount": 0,
"state": {
"running": {
"startedAt": "2019-06-13T14:20:07Z"
}
}
}
],
"hostIP": "192.168.0.10",
"phase": "Running",
"podIP": "172.16.1.9",
"qosClass": "BestEffort",
"startTime": "2019-06-13T14:19:17Z"
}
}
查看
[root@k8s-master ~]# kubectl get pods -n appblog
No resources found.
以上,pod创建与删除功能开发完成。
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/23/pod-creation-and-deletion-for-kubernetes-api-microservice-development/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

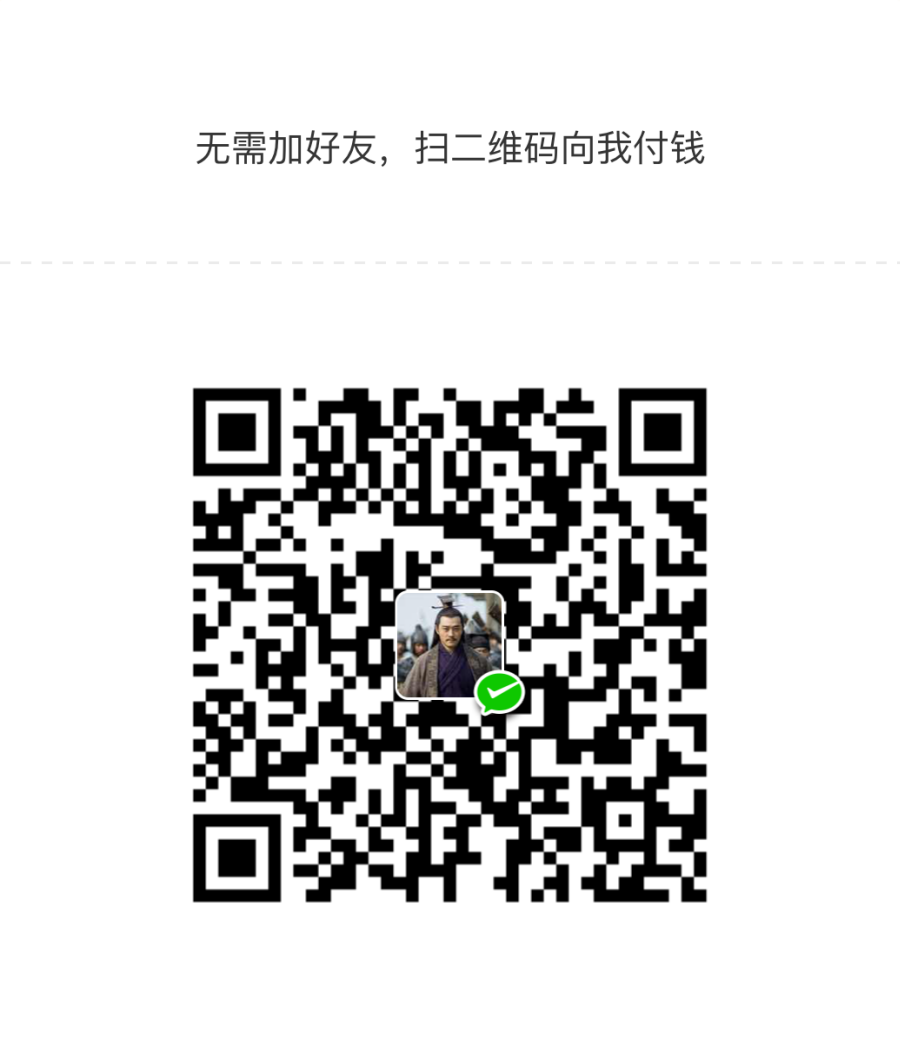

共有 0 条评论