JDK动态代理的原理
Proxy介绍
之前在研究hook的时候,使用到了Proxy动态代理。它是来自JDK的一个类:
package java.lang.reflect;
public class Proxy implements java.io.Serializable {
关键方法是:
@CallerSensitive
public static Object newProxyInstance(ClassLoader loader,
Class<?>[] interfaces,
InvocationHandler h)
throws IllegalArgumentException
{
}
使用起来很简单:
/**
* 获得动态代理
*
* @return
*/
public Object getProxyInstance() {
return Proxy.newProxyInstance(
realInstance.getClass().getClassLoader(),
realInstance.getClass().getInterfaces(),
this);
//那么这个对象到底是如何创建出来的呢?
}
只是一句话而已,就能得到一个接口的动态代理。
动态代理实现
只是一个很简单的类DynamicProxyInstance.java
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
/**
* 动态代理对象
* 所谓,动态代理,它的优势,就是我使用之后,获取代理对象,不再依赖外部传入的接口,外部接口变化,我只需要改变真实对象的实例即可
*/
public class DynamicProxyInstance implements InvocationHandler {
// 动态代理,这里不限制具体使用的接口是什么,而是使用Object表示真实对象
private Object realInstance;
public void init(Object realInstance) {
this.realInstance = realInstance;
}
/**
* 获得动态代理
*
* @return
*/
public Object getProxyInstance() {
return Proxy.newProxyInstance(realInstance.getClass().getClassLoader(), realInstance.getClass().getInterfaces(),
this);
// 那么这个对象到底是如何创建出来的呢?
}
/**
* 售前
*/
private void doBefore() {
System.out.println("售前");
}
/**
* 售后
*/
private void doAfter() {
System.out.println("售后");
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
doBefore();
Object res = method.invoke(realInstance, args);
doAfter();
return res;
}
public static void main(String[] args) {
// 动态代理和静态代理的唯一区别,就是在获取代理对象的时候,静态代理获取的是一个不可变功能的对象,一旦获取到,那么它的功能也就确定了
// 而,动态代理
DynamicProxyInstance instance = new DynamicProxyInstance();
instance.init(new RealInstance());
CommInterface commInterface = (CommInterface) instance.getProxyInstance();
// 先看看这个对象在debug的时候,是怎么样的一个对象引用
commInterface.buyManTools(30);
// 现在,出现了一个购买女性用品的接口,和它的实现类,也要用代理来访问
// 这样做
instance.init(new RealInstance2());
CommInterface2 commInterface2 = (CommInterface2) instance.getProxyInstance();
commInterface2.buyWomenTools(60);
// 看到了吧,同样的一个代理对象,可以实现多个真实对象的代理功能,而不是像静态代理一样,需要改动代理类
}
}
其他关联的类和接口是:
/**
* 公共接口,统一定义代理类和被代理类的行为
*/
public interface CommInterface {
/**
* 购买情趣娃娃
*
* @param size 娃娃的尺寸
*/
abstract void buyManTools(int size);
}
/**
* 公共接口,统一定义代理类和被代理类的行为
*/
public interface CommInterface2 {
/**
* 购买情趣娃娃
*
* @param size 娃娃的尺寸
*/
abstract void buyWomenTools(int size);
}
/**
* 真实对象
*/
public class RealInstance implements CommInterface {
@Override
public void buyManTools(int size) {
System.out.println("购买男性用品:size" + size);
}
}
/**
* 真实对象
*/
public class RealInstance2 implements CommInterface2 {
@Override
public void buyWomenTools(int size) {
System.out.println("购买女性用品::size" + size);
}
}
OK,代码贴完了,现在开始实例讲解:
上面的代码,是在模拟XX用品销售,男用、女用的分别用两个接口来定义,为了定义这两个功能,我用了两个接口,并且用两个实现类分别实现了这两个接口。DynamicProxyInstance.java
则是代理类,外界(也就是这里的static void main
函数)通过该代理类来进行购买动作
请看这段代码,它是new
出了一个proxy
的Object
,并且return
出去
public Object getProxyInstance() {
return Proxy.newProxyInstance(realInstance.getClass().getClassLoader(), realInstance.getClass().getInterfaces(),
this);
// 那么这个对象到底是如何创建出来的呢?
}
而,拿到这个对象之后,强转为了CommInterface
// 先看看这个对象在debug的时候,是怎么样的一个对象引用
CommInterface commInterface = (CommInterface) instance.getProxyInstance();
commInterface.buyManTools(30);
为什么它可以强转为CommInterface
?因为instance.init(new RealInstance())
,这一句当中RealInstance
是CommIntance
的子类。从结果上来看,通过getInstance
方法拿到的Object
,直接就具备realInstance
的功能。那这个Object
到底是什么东西?它是如何生成的?
回到getInstance()
方法,通过debug,我们发现
CommInterface commInterface = (CommInterface) instance.getProxyInstance();
它是一个名为$Proxy0
的类的实例,其真实类型是RealInstance
,$Proxy0
名字很诡异,但是先别想这么多,继续往下看
CommInterface2 commInterface2 = (CommInterface2) instance.getProxyInstance();
这会又变成了$Proxy1
,其真实类型是RealInstance2
从0到1,看上去像是有一个整形序列在参与它的命名,也就是说,如果我们再来一次,肯定就是$Proxy2
那$Proxy
+ 1个数字,这个类是如何生成的?在我们的代码里面命名没有去定义它啊?
进入源码:Proxy.java
的newProxyInstance
方法
@CallerSensitive
public static Object newProxyInstance(ClassLoader loader,
Class<?>[] interfaces,
InvocationHandler h)
throws IllegalArgumentException {
Objects.requireNonNull(h);
final Class<?>[] intfs = interfaces.clone();
final SecurityManager sm = System.getSecurityManager();
if (sm != null) {
checkProxyAccess(Reflection.getCallerClass(), loader, intfs);
}
/*
* Look up or generate the designated proxy class.
*/
Class<?> cl = getProxyClass0(loader, intfs);
这里有3个参数:
- 第一个是
类加载器ClassLoader
- 第二个是
接口(它的作用是定义这个动态代理类的行为)
- 第三个是
InvocationHanlder实例,它的作用是用来对 被代理对象进行功能扩展
newProxyInstance
方法往下看:Class<?> cl = getProxyClass0(loader, intfs);
,这里是不是可能生成$Proxy1
这种class
的地方?进去:
private static Class<?> getProxyClass0(ClassLoader loader,
Class<?>... interfaces) {
if (interfaces.length > 65535) {
throw new IllegalArgumentException("interface limit exceeded");
}
// If the proxy class defined by the given loader implementing
// the given interfaces exists, this will simply return the cached copy;
// otherwise, it will create the proxy class via the ProxyClassFactory
return proxyClassCache.get(loader, interfaces);
}
观察一下这个proxyClassCache
对象,它的类型是:WeakCache<ClassLoader, Class<?>[], Class<?>>
它是一个泛型类:final class WeakCache<K, P, V>
3个泛型参数。
/**
* a cache of proxy classes
*/
private static final WeakCache<ClassLoader, Class<?>[], Class<?>>
proxyClassCache = new WeakCache<>(new KeyFactory(), new ProxyClassFactory());
进入proxyClassCache.get(loader, interfaces)
public V get(K key, P parameter) { //关注点1:入参
Objects.requireNonNull(parameter);
expungeStaleEntries();
Object cacheKey = CacheKey.valueOf(key, refQueue);
// lazily install the 2nd level valuesMap for the particular cacheKey
ConcurrentMap<Object, Supplier<V>> valuesMap = map.get(cacheKey);
if (valuesMap == null) {
ConcurrentMap<Object, Supplier<V>> oldValuesMap
= map.putIfAbsent(cacheKey,
valuesMap = new ConcurrentHashMap<>());
if (oldValuesMap != null) {
valuesMap = oldValuesMap;
}
}
// create subKey and retrieve the possible Supplier<V> stored by that
// subKey from valuesMap
Object subKey = Objects.requireNonNull(subKeyFactory.apply(key, parameter)); //关注点2:入参的使用
Supplier<V> supplier = valuesMap.get(subKey);
Factory factory = null;
while (true) {
if (supplier != null) {
// supplier might be a Factory or a CacheValue<V> instance
V value = supplier.get();
if (value != null) {
return value; //关注点3:函数返回值
}
}
它的作用就是,通过K
和P
,得到V
,也就是通过ClassLoader
和Class<?>[]
得到Class<?>
。ClassLoader
是类加载器,用来创建Class
对象的,Class<?>[]
其实就是接口数组,Class<?>
是最终得到的Class对象。
之前我们探索的就是$Proxy0
、$Proxy1
这个类的来源,那么到底是不是来自于这里呢?
当我们探究源码中的一个方法的时候,关注3个点:
- 入参类型(这里的入参类型是泛型,具体类型要看这个当前对象在创建的时候,泛型具体化使用的是什么类)
- 使用参数的地方(我们入参传递进来,自然要产生作用,那么直接关心入参使用的代码即可)
- 方法返回值,(上面的这个方法有返回值,类型是泛型,也去看初始化对象的时候使用的
V
是什么类型,然后方法里面返回的value,就是我们最终需要的对象)
关注subkeyFactory.apply
,在Proxy中,定义WeakCache的时候,就new
了一个KeyFactory
和一个ProxyClassFactory
传进去,分别作为WeakCache
的subkeyFactory
成员和valueFactory
成员
final class WeakCache<K, P, V> {
public WeakCache(BiFunction<K, P, ?> subKeyFactory,
BiFunction<K, P, V> valueFactory) {
this.subKeyFactory = Objects.requireNonNull(subKeyFactory);
this.valueFactory = Objects.requireNonNull(valueFactory);
}
上面,我们只使用了subkeyFactory.apply
,它走的是这个过程:
public class Proxy implements java.io.Serializable {
/**
* A function that maps an array of interfaces to an optimal key where
* Class objects representing interfaces are weakly referenced.
*/
private static final class KeyFactory
implements BiFunction<ClassLoader, Class<?>[], Object>
{
@Override
public Object apply(ClassLoader classLoader, Class<?>[] interfaces) {
switch (interfaces.length) {
case 1: return new Key1(interfaces[0]); // the most frequent
case 2: return new Key2(interfaces[0], interfaces[1]);
case 0: return key0;
default: return new KeyX(interfaces);
}
}
}
根据参数interfaces.length
的不同,返回不同的Key对象。
// create subKey and retrieve the possible Supplier<V> stored by that
// subKey from valuesMap
Object subKey = Objects.requireNonNull(subKeyFactory.apply(key, parameter)); //拿到key对象
Supplier<V> supplier = valuesMap.get(subKey); //使用key对象,尝试从valuesMap中取出valueFactory
Factory factory = null;
while (true) { //无限循环
if (supplier != null) { //如果前面取到的supplier不是空
// supplier might be a Factory or a CacheValue<V> instance
V value = supplier.get(); //那就从supplier中取出value,并且返回出去
if (value != null) {
return value;
}
}
// else no supplier in cache
// or a supplier that returned null (could be a cleared CacheValue
// or a Factory that wasn't successful in installing the CacheValue)
// lazily construct a Factory
if (factory == null) { //如果到了这一步,说明前面没有return,建立一个工厂
factory = new Factory(key, parameter, subKey, valuesMap);
}
if (supplier == null) {
supplier = valuesMap.putIfAbsent(subKey, factory); //putIfAbsent的作用相当于,一个map,如果存了subKey对应的value,就返回这个value,如果没有存,就存进去
if (supplier == null) {
// successfully installed Factory
supplier = factory;
}
// else retry with winning supplier
} else {
if (valuesMap.replace(subKey, supplier, factory)) {
// successfully replaced
// cleared CacheEntry / unsuccessful Factory
// with our Factory
supplier = factory;
} else {
// retry with current supplier
supplier = valuesMap.get(subKey);
}
}
}
上面这段代码的注释如果看懂了的话,就基本明白了,它这里就是建了一个无限循环检测机制,最终结果都是要从supplier
中get
一个value
返回出去,如果supplier
是空,那就建一个Factory
赋值给supplier
(其实Factory
是继承至Supplier
的)
那么下一步,我们的关注点转移到,当supplier
为空的时候,源码是如何建立一个Factory
作为它的实例的?
// lazily construct a Factory
if (factory == null) {
factory = new Factory(key, parameter, subKey, valuesMap);
}
进入Factory
,它实现Supplier
接口,这个接口中只有一个方法get
,看看这个get
方法是如何实现的
private final class Factory implements Supplier<V> {
private final K key;
private final P parameter;
private final Object subKey;
private final ConcurrentMap<Object, Supplier<V>> valuesMap;
Factory(K key, P parameter, Object subKey,
ConcurrentMap<Object, Supplier<V>> valuesMap) {
this.key = key;
this.parameter = parameter;
this.subKey = subKey;
this.valuesMap = valuesMap;
}
@Override
public synchronized V get() { // serialize access
// re-check
Supplier<V> supplier = valuesMap.get(subKey); //看来这里有缓存机制
if (supplier != this) {
// something changed while we were waiting:
// might be that we were replaced by a CacheValue
// or were removed because of failure ->
// return null to signal WeakCache.get() to retry
// the loop
return null;
}
// else still us (supplier == this)
// create new value
V value = null;
try {
value = Objects.requireNonNull(valueFactory.apply(key, parameter)); //这里通过valueFactory.apply拿到value
} finally {
if (value == null) { // remove us on failure
valuesMap.remove(subKey, this);
}
}
// the only path to reach here is with non-null value
assert value != null;
// wrap value with CacheValue (WeakReference)
CacheValue<V> cacheValue = new CacheValue<>(value);
// put into reverseMap
reverseMap.put(cacheValue, Boolean.TRUE);
// try replacing us with CacheValue (this should always succeed)
if (!valuesMap.replace(subKey, this, cacheValue)) {
throw new AssertionError("Should not reach here");
}
// successfully replaced us with new CacheValue -> return the value
// wrapped by it
return value; //最终返回出去的对象
}
}
所以最终,我们还要进入valueFactory
中去看看它的apply
是如何实现的:
public class Proxy implements java.io.Serializable {
private static final class ProxyClassFactory
implements BiFunction<ClassLoader, Class<?>[], Class<?>>
{
// prefix for all proxy class names
private static final String proxyClassNamePrefix = "$Proxy"; //类名前缀
// next number to use for generation of unique proxy class names
private static final AtomicLong nextUniqueNumber = new AtomicLong(); //全局计数器,计算下一个代理类对象应该以什么数字命名
@Override
public Class<?> apply(ClassLoader loader, Class<?>[] interfaces) {
...
String proxyPkg = null; // package to define proxy class in
int accessFlags = Modifier.PUBLIC | Modifier.FINAL;
/*
* Record the package of a non-public proxy interface so that the
* proxy class will be defined in the same package. Verify that
* all non-public proxy interfaces are in the same package.
*/
for (Class<?> intf : interfaces) {
int flags = intf.getModifiers();
if (!Modifier.isPublic(flags)) {
accessFlags = Modifier.FINAL;
String name = intf.getName();
int n = name.lastIndexOf('.');
String pkg = ((n == -1) ? "" : name.substring(0, n + 1));
if (proxyPkg == null) {
proxyPkg = pkg;
} else if (!pkg.equals(proxyPkg)) {
throw new IllegalArgumentException(
"non-public interfaces from different packages");
}
}
}
if (proxyPkg == null) {
// if no non-public proxy interfaces, use com.sun.proxy package
proxyPkg = ReflectUtil.PROXY_PACKAGE + ".";
}
/*
* Choose a name for the proxy class to generate.
*/
long num = nextUniqueNumber.getAndIncrement();
String proxyName = proxyPkg + proxyClassNamePrefix + num;
/*
* Generate the specified proxy class.
*/
byte[] proxyClassFile = ProxyGenerator.generateProxyClass(
proxyName, interfaces, accessFlags);
try {
return defineClass0(loader, proxyName,
proxyClassFile, 0, proxyClassFile.length);
} catch (ClassFormatError e) {
/*
* A ClassFormatError here means that (barring bugs in the
* proxy class generation code) there was some other
* invalid aspect of the arguments supplied to the proxy
* class creation (such as virtual machine limitations
* exceeded).
*/
throw new IllegalArgumentException(e.toString());
}
}
}
这段代码太复杂,这里只贴出关键点(依然和之前一样,入参,使用入参的地方,返回值),这次我们从返回值开始反过来推断:
try {
return defineClass0(loader, proxyName,
proxyClassFile, 0, proxyClassFile.length);
} catch (ClassFormatError e) {
源码直接返回了一个defineClass0(.....)
,点进去一看,居然是一个native方法,那么探索只能到头了。当然,从结果上来看,它是生成了一个像这样$Proxy0
的Class
代理对象,那么代理Class
对象的特征,就只能从参数值来判断了,一个一个参数看:
private static native Class<?> defineClass0(ClassLoader loader, String name,
byte[] b, int off, int len);
ClassLoader loader
类加载器String name
类名byte[] b, int off, int len
字节码IO流相关的参数
反推,类名是如何来的?IO流字节码的3个参数是干什么用的?
/*
* Choose a name for the proxy class to generate.
*/
long num = nextUniqueNumber.getAndIncrement();
String proxyName = proxyPkg + proxyClassNamePrefix + num; //包名 类名前缀$Proxy 序号(AtomicLong nextUniqueNumber自增得到)
/*
* Generate the specified proxy class.
*/
byte[] proxyClassFile = ProxyGenerator.generateProxyClass( //字节码
proxyName, interfaces, accessFlags); //类名 接口 接口修饰符
try {
return defineClass0(loader, proxyName,
proxyClassFile, 0, proxyClassFile.length);
} catch (ClassFormatError e) {
/*
* A ClassFormatError here means that (barring bugs in the
* proxy class generation code) there was some other
* invalid aspect of the arguments supplied to the proxy
* class creation (such as virtual machine limitations
* exceeded).
*/
throw new IllegalArgumentException(e.toString());
}
而上面的字节数组byte[] proxyClassFile
其实就是定义了这个代理类的行为,它应该能包含什么样的方法,这些方法就是当初传进来的interfaces
决定的。
OK,探究完了,代理类的生成已经查完,那么回到起点Class<?> cl = getProxyClass0(loader, intfs)
,我们拿到这个代理类对象Class<?> cl
之后能干啥? 肯定是使用它来反射创建代理对象啊
public static Object newProxyInstance(ClassLoader loader,
Class<?>[] interfaces,
InvocationHandler h)
throws IllegalArgumentException
{
...
/*
* Look up or generate the designated proxy class.
*/
Class<?> cl = getProxyClass0(loader, intfs); //前面拿到的代理类对象$Proxy0
/*
* Invoke its constructor with the designated invocation handler.
*/
try {
if (sm != null) {
checkNewProxyPermission(Reflection.getCallerClass(), cl);
}
final Constructor<?> cons = cl.getConstructor(constructorParams); //取得构造函数
final InvocationHandler ih = h; //这是外部传进来的InvocationHandler对象
if (!Modifier.isPublic(cl.getModifiers())) {
AccessController.doPrivileged(new PrivilegedAction<Void>() {
public Void run() {
cons.setAccessible(true);
return null;
}
});
}
return cons.newInstance(new Object[]{h}); //这里反射创建一个代理类对象并return出去,注意参数h
} catch (IllegalAccessException|InstantiationException e) {
throw new InternalError(e.toString(), e);
} catch (InvocationTargetException e) {
Throwable t = e.getCause();
if (t instanceof RuntimeException) {
throw (RuntimeException) t;
} else {
throw new InternalError(t.toString(), t);
}
} catch (NoSuchMethodException e) {
throw new InternalError(e.toString(), e);
}
}
这里有一个关键点,参数h
,它是我们从外部传入的InvocationHandler
对象,这个对象定义了我们对被代理类的方法增强,即我们可以在被代理类的指定方法(或者所有方法)执行的过程中,加入前置或者后置过程,还可以设置条件,根据条件决定被代理类的方法要不要执行。那么它是如何发挥作用的呢?
/*
* Generate the specified proxy class.
*/
byte[] proxyClassFile = ProxyGenerator.generateProxyClass( //字节码
proxyName, interfaces, accessFlags); //类名 接口 接口修饰符
try {
return defineClass0(loader, proxyName,
proxyClassFile, 0, proxyClassFile.length);
} catch (ClassFormatError e) {
还记得这个字节码IO流相关的参数么,其实如果把这个byte[]
通过IO流输出到本地文件,其实就是一个.class
文件,反编译出来,就是一个.java
文件,类似下面的:
public final class $Proxy0
extends Proxy //派生自Proxy类
implements AaFactory //实现了业务接口
{
private static Method m1; //各种方法
private static Method m8;
private static Method m3;
private static Method m2;
private static Method m6;
private static Method m5;
private static Method m7;
private static Method m9;
private static Method m0;
private static Method m4;
public final void saleManTools(String paramString)
throws
{
try
{
this.h.invoke(this, m3, new Object[] { paramString }); //m3是其中一个代理方法
return;
}
这里保存了来自外部接口的各种方法(命名也是很耿直 m1,m2.....),并且这个saleManTools
,就是我们demo中的接口的一个方法,它反射执行了这个方法,这里的h
,其实就是我们最初给的invocationHandler
对象:
public class DynamicProxyInstance implements InvocationHandler {
/**
* 获得动态代理
*
* @return
*/
public Object getProxyInstance() {
return Proxy.newProxyInstance(realInstance.getClass().getClassLoader(), realInstance.getClass().getInterfaces(),
this); //this即InvocationHandler对象
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
doBefore();
Object res = method.invoke(realInstance, args);
doAfter();
return res;
}
当我们调用代理对象的业务方法时,其实也执行了invocationHandler
的invoke
方法,那么对被代理对象的方法的增强或者限制,我们就可以在重写invoke
时随意处理了。
结语
就写到这里吧,做个总结,java.lang.reflect.Proxy
是JDK提供的动态代理类,我们探索过程中,至少发现了2处使用反射的地方,一处是 反射构造函数,然后执行它,创建代理类对象的过程,一处是使用代理类对象,反射创建代理对象的过程(代理类对象和代理对象是不一样的)。
使用了反射,效率自然比不上静态代理,但是它能保证真实对象无限扩展的时候,代理类不用做修改,只需要在创建代理类的时候,传入不同的真实对象即可,这种做法才符合程序设计的开闭原则,对扩展开放,对修改关闭。
我们去使用一个类,一定会先和获得这个类的class
对象,利用Proxy
可以动态去创建Proxy0
,proxy1
这样子的class
对象。然后才利用这个对象反射执行构造函数去创建proxy0
对象。
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/25/principle-of-jdk-dynamic-proxy/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

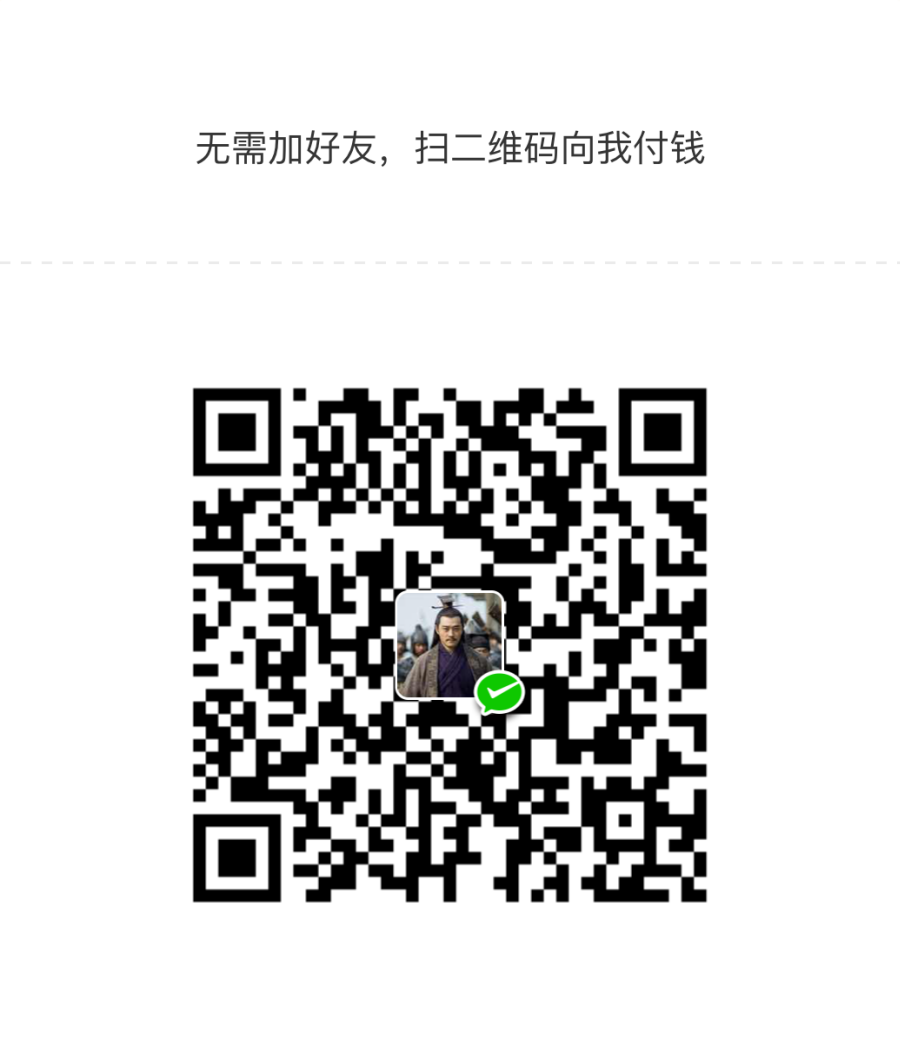

共有 0 条评论