Shopify接口开发(PHP)
Shopify简介
Shopify是由托比亚斯·卢克创办的加拿大电子商务软件开发商,总部位于加拿大首都渥太华,其提供的服务软件Shopify是一个SaaS领域的购物车系统,适合跨境电商建立独立站,用户支付一定费用即可在其上利用各种主题/模板建立自己的网上商店。
文档地址
- 授权 OAuth: https://shopify.dev/tutorials/authenticate-with-oauth
- 使用接口 REST Admin API reference: https://shopify.dev/docs/admin-api/rest/reference
- API(Ruby & Node.js & PHP): https://shopify.dev/tools/libraries/admin-api
接入示例
$shop = 'xxx'; //店铺名,指的是 后台登陆地址栏内 xxx.myshopify.com 这个 xxx
$api_key = "xxx"; //app key 在开发者平台中创建app就会生成
//需要获取的权限
$scopes = "read_locations,read_content,write_content,read_themes,write_themes,read_products,write_products,read_product_listings,read_collection_listings,read_customers,write_customers,read_orders,write_orders,read_draft_orders,write_draft_orders,read_script_tags,write_script_tags,read_fulfillments,write_fulfillments,read_shipping,write_shipping,read_analytics,read_checkouts,write_checkouts,read_reports,write_reports,read_price_rules,write_price_rules,read_marketing_events,write_marketing_events,read_resource_feedbacks,write_resource_feedbacks,read_locations,read_inventory,write_inventory";
//返回地址 这个可以任意填写
$redirect_uri = "";
//构建请求地址
$install_url = "https://" . $shop . ".myshopify.com/admin/oauth/authorize?client_id=" . $api_key . "&scope=" . $scopes . "&redirect_uri=" . urlencode($redirect_uri);
//请求地址生成后可直接使用 GET 方式发送
//进入shopify授权界面,此时输入用户名密码,后点击授权
//返回地址上会有 code=xxxx
//接下来获取token值
//构建请求信息
$request_headers = array(
"Content-type" => "application/json", // 告诉Shopify我们期待JSON格式的回复
"client_id" => "daeaac41584625af84a5707f708336f6", // 创建app时产生
"client_secret" => "1f73927bbefec664c142fd22e118de59", // 创建app时产生
"code" => $code // 上面返回的code值
);
//发送请求,获得返回值
$shopify_response = $this->shopify_call(NULL, $shop, "/admin/oauth/access_token", $request_headers, 'POST', $request_headers);
//解析json字符串
$shopify_response = json_decode($shopify_response['response'], TRUE);
//示例
//订单接口
$create_time_from_date=date('Y-m-d',$create_time_from).'T'.date('H:i:s',$create_time_from);
$opt=array(
"limit" => 200,
"page" => $page,
"financial_status" => 'paid',
"updated_at_min" => $create_time_from_date,
);
$orders = $this-> shopify_call($token, $shop, "/admin/orders.json", $opt, 'GET');
//产品创建接口
$opt = array();
$insert = array();
$insert['title'] = '';
$insert['body_html'] ='';
$insert['vendor'] = '';
$insert['product_type'] = '';
$insert['tags'] = '';
//还有可以添加很多参数
$opt['product'] = $insert;
$product = $this->shopify_call($token,$shop,"/admin/api/2019-07/products.json",$opt,'POST',array(0=>'Content-Type: application/json'));
//使用的基础函数
//$token 上方获取的token值
//$shop 同上方相同
//$api_endpoint 接口路径
//$query 接口参数
//$method 访问方式
//$request_headers 头部信息
function shopify_call($token, $shop, $api_endpoint, $query = array(), $method = 'GET', $request_headers = array()) {
// Build URL
$url = "https://" . $shop . ".myshopify.com" . $api_endpoint;
if (!is_null($query) && in_array($method, array('GET', 'DELETE'))) $url = $url . "?" . http_build_query($query);
// Configure cURL
$curl = curl_init($url);
curl_setopt($curl, CURLOPT_HEADER, TRUE);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt($curl, CURLOPT_FOLLOWLOCATION, TRUE);
curl_setopt($curl, CURLOPT_MAXREDIRS, 3);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE);
// curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, 3);
// curl_setopt($curl, CURLOPT_SSLVERSION, 3);
curl_setopt($curl, CURLOPT_USERAGENT, 'My New Shopify App v.1');
curl_setopt($curl, CURLOPT_CONNECTTIMEOUT, 30);
curl_setopt($curl, CURLOPT_TIMEOUT, 30);
curl_setopt($curl, CURLOPT_CUSTOMREQUEST, $method);
// Setup headers
$request_headers[] = "";
if (!is_null($token)) $request_headers[] = "X-Shopify-Access-Token: " . $token;
curl_setopt($curl, CURLOPT_HTTPHEADER, $request_headers);
if ($method != 'GET' && in_array($method, array('POST', 'PUT'))) {
if (is_array($query)) {
$fquery = http_build_query($query);
if($request_headers[0]=='Content-Type: application/json')
$fquery=json_encode($query);
}
curl_setopt ($curl, CURLOPT_POSTFIELDS, $fquery);
}
// Send request to Shopify and capture any errors
$response = curl_exec($curl);
$response = str_replace("HTTP/1.1 100 Continue\r\r",'', $response);
$response = str_replace("HTTP/1.1 100 Continue\r\n\r\n",'', $response);
$response = str_replace("HTTP/1.1 100 Continue\n\n",'', $response);
$error_number = curl_errno($curl);
$error_message = curl_error($curl);
// Close cURL to be nice
curl_close($curl);
// Return an error is cURL has a problem
if ($error_number) {
return $error_message;
} else {
// No error, return Shopify's response by parsing out the body and the headers
$response = preg_split("/\r\n\r\n|\n\n|\r\r/", $response, 2);
// Convert headers into an array
$headers = array();
$header_data = explode("\n",$response[0]);
$headers['status'] = $header_data[0]; // Does not contain a key, have to explicitly set
array_shift($header_data); // Remove status, we've already set it above
foreach($header_data as $part) {
$h = explode(":", $part);
$headers[trim($h[0])] = trim($h[1]);
}
// Return headers and Shopify's response
return array('headers' => $headers, 'response' => $response[1]);
}
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/26/shopify-api-development-php/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

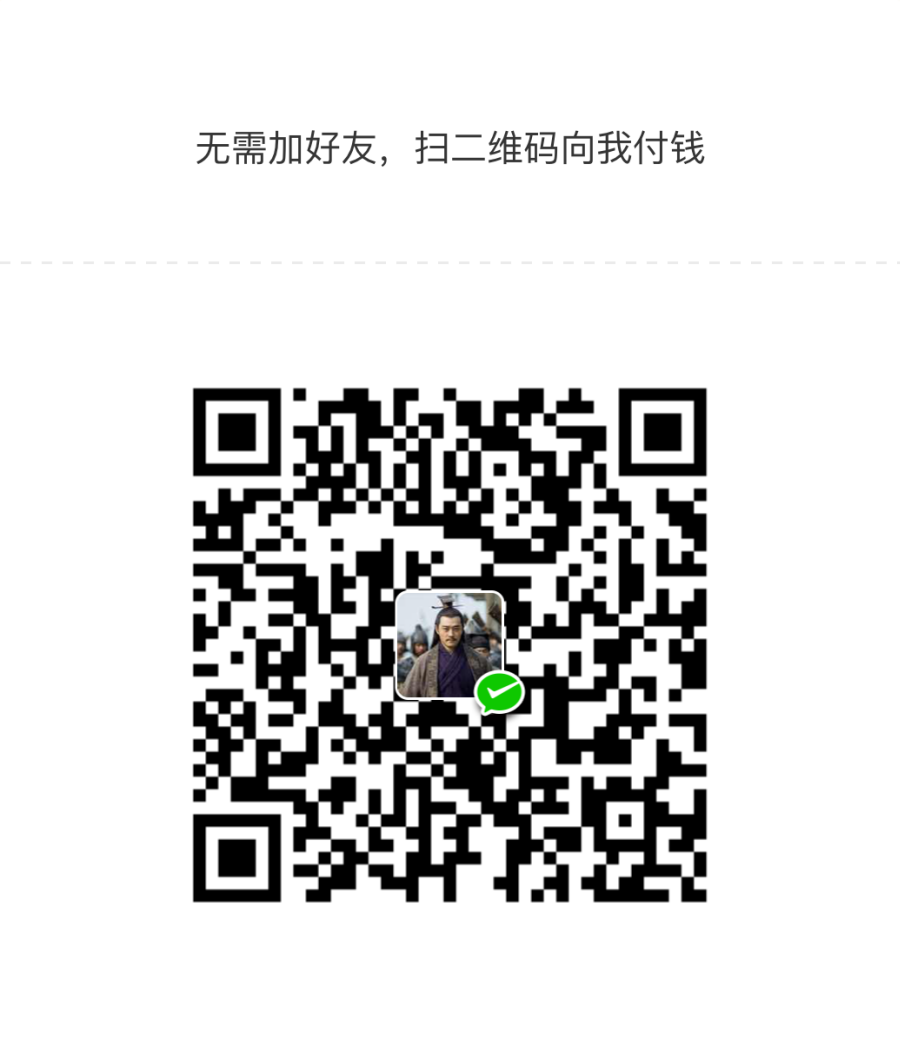
Shopify接口开发(PHP)
Shopify简介
Shopify是由托比亚斯·卢克创办的加拿大电子商务软件开发商,总部位于加拿大首都渥太华,其提供的服务软件Shopify是一个SaaS领域的购物车系统,适……

文章目录
关闭
共有 0 条评论