Java中将Word生成缩略图
解决思路
1、先将word生成pdf,这个采用openoffice或者jacob
2、然后将pdf生成图片
注:需要安装OpenOffice依赖,并启动
soffice
服务
依赖引入
需要的jar包是pdfrenderer.jar
和jodconverter-2.2.2.jar
包,如果使用jacob
还得加入jacob.jar
注:Maven上
pdfrenderer.jar
不能下载,jodconverter.jar
只能下载2.2.1版本的,两者均需要手动下载或部署至私服
- JODConverter下载地址:https://sourceforge.net/projects/jodconverter/files/JODConverter/
- PDFRenderer下载地址:https://artifacts.alfresco.com/nexus/content/repositories/public/com/sun/pdfview/pdfrenderer/
<!-- https://mvnrepository.com/artifact/com.artofsolving/jodconverter -->
<dependency>
<groupId>com.artofsolving</groupId>
<artifactId>jodconverter</artifactId>
<version>2.2.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.sun.pdfview/pdfrenderer -->
<dependency>
<groupId>com.sun.pdfview</groupId>
<artifactId>pdfrenderer</artifactId>
<version>0.9.1-patched</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.openoffice/ridl -->
<dependency>
<groupId>org.openoffice</groupId>
<artifactId>ridl</artifactId>
<version>4.1.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.slf4j/slf4j-api -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.8.0</version>
</dependency>
代码实现
具体代码如下:
public static void main(String[] args) throws IOException {
File officeFile = new File("D:/test.doc");
File pdfFile = new File("D:/test.pdf");
officeToPdf(officeFile, pdfFile);
pdfToJPG("D:/test.pdf", "D:/");
}
private static void officeToPdf(File officeFile, File pdfFile) {
OpenOfficeConnection connection = new SocketOpenOfficeConnection("127.0.0.1", 8100);
try {
connection.connect();
DocumentConverter converter = new OpenOfficeDocumentConverter(
connection);
converter.convert(officeFile, pdfFile);
} catch (ConnectException e) {
e.printStackTrace();
} finally {
// close the connection
connection.disconnect();
}
}
// 将PDF格式的文件转换为JPG格式的文件
private static void pdfToJPG(String pdfPath, String imagePath) throws IOException {
// load a pdf from a byte buffer
File file = new File(pdfPath);
RandomAccessFile raf = new RandomAccessFile(file, "r");
FileChannel channel = raf.getChannel();
//这句代码通道建立了map映射,如果要删除file那么得接触映射
ByteBuffer buf = channel.map(FileChannel.MapMode.READ_ONLY, 0,
channel.size());
PDFFile pdffile = new PDFFile(buf);
int totalPage = pdffile.getNumPages();
for (int i = 1; i <= totalPage; i++) {
if (i == 1) {
// draw the first page to an image
// 以图片的形式来描绘首页
PDFPage page = pdffile.getPage(i);
Rectangle rect = new Rectangle(0, 0, (int) page.getBBox()
.getWidth(), (int) page.getBBox().getHeight());
// generate the image
// 生成图片
Image img = page.getImage(rect.width, rect.height, // width & height
rect, // clip rect
null, // null for the ImageObserver
true, // fill background with white
true // block until drawing is done
);
BufferedImage tag = new BufferedImage(rect.width, rect.height,
BufferedImage.TYPE_INT_RGB);
tag.getGraphics().drawImage(img.getScaledInstance(rect.width, rect.height, Image.SCALE_SMOOTH), 0, 0, rect.width, rect.height,
null);
FileOutputStream out = new FileOutputStream(imagePath + File.separator + pdfPath.substring(pdfPath.lastIndexOf("/") + 1, pdfPath.lastIndexOf("."))
+ ".jpg"); // 输出到文件流
JPEGImageEncoder encoder = JPEGCodec.createJPEGEncoder(out);
encoder.encode(tag); // JPEG编码
// 关闭输出流
out.close();
break;
}
}
buf.clear();
channel.close();
raf.close();
unmap(buf);
//file.delete();
}
//解除map映射
public static <T> void unmap(final Object buffer) {
AccessController.doPrivileged(new PrivilegedAction<T>() {
@Override
public T run() {
try {
Method getCleanerMethod = buffer.getClass().getMethod("cleaner", new Class[0]);
getCleanerMethod.setAccessible(true);
Cleaner cleaner = (sun.misc.Cleaner) getCleanerMethod.invoke(buffer, new Object[0]);
cleaner.clean();
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
});
}
需要注意的是,生成完图片后将pdf删除,但是删除失败,经过大牛的指点加上了unmap
就ok了,故写下来分享给大家。
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/04/01/generating-thumbnails-for-word-in-java/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

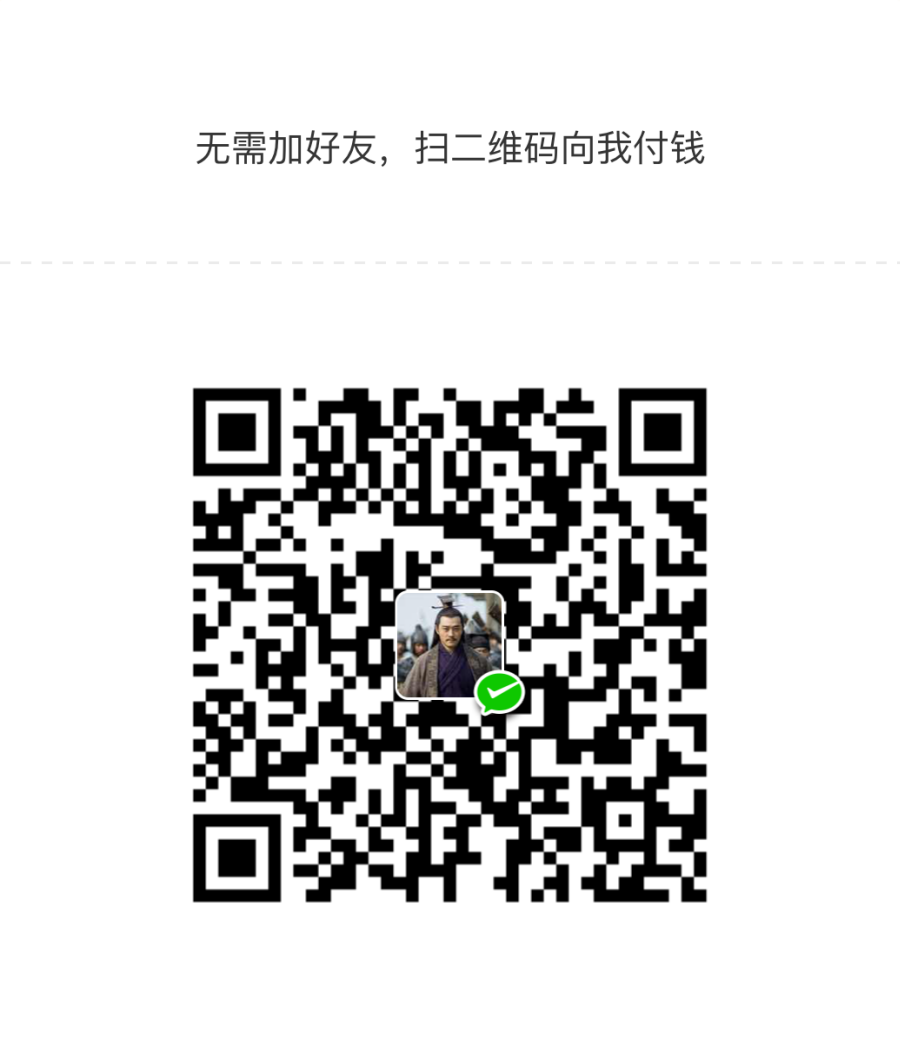
Java中将Word生成缩略图
解决思路
1、先将word生成pdf,这个采用openoffice或者jacob
2、然后将pdf生成图片
注:需要安装OpenOffice依赖,并启动soffice服务
依赖引入
需要的jar包……

文章目录
关闭
共有 0 条评论