Spring Boot 接收微信支付通知参数
微信支付通知参数
首先明确微信支付通知参数是xml格式,示例如下:
<xml>
<openid><![CDATA[sandboxopenid]]></openid>
<trade_type><![CDATA[NATIVE]]></trade_type>
<coupon_fee><![CDATA[3]]></coupon_fee>
<cash_fee_type><![CDATA[CNY]]></cash_fee_type>
<nonce_str><![CDATA[20200110152332175]]></nonce_str>
<time_end><![CDATA[20200110152337]]></time_end>
<err_code_des><![CDATA[SUCCESS]]></err_code_des>
<return_code><![CDATA[SUCCESS]]></return_code>
<mch_id><![CDATA[128875980]]></mch_id>
<settlement_total_fee><![CDATA[100]]></settlement_total_fee>
<sign><![CDATA[2767F6B8A2D87850572D66C5737377E5]]></sign>
<cash_fee><![CDATA[99]]></cash_fee>
<coupon_id_1><![CDATA[67890]]></coupon_id_1>
<coupon_type_0><![CDATA[CASH]]></coupon_type_0>
<coupon_id_0><![CDATA[123456]]></coupon_id_0>
<coupon_fee_0><![CDATA[1]]></coupon_fee_0>
<coupon_fee_1><![CDATA[2]]></coupon_fee_1>
<is_subscribe><![CDATA[Y]]></is_subscribe>
<return_msg><![CDATA[OK]]></return_msg>
<fee_type><![CDATA[CNY]]></fee_type>
<bank_type><![CDATA[CMC]]></bank_type>
<attach><![CDATA[pay-test]]></attach>
<device_info><![CDATA[sandbox]]></device_info>
<out_trade_no><![CDATA[wx20200110152332175]]></out_trade_no>
<coupon_type_1><![CDATA[NO_CASH]]></coupon_type_1>
<result_code><![CDATA[SUCCESS]]></result_code>
<total_fee><![CDATA[102]]></total_fee>
<appid><![CDATA[wx28f9d88adc6a2f8c]]></appid>
<coupon_count><![CDATA[2]]></coupon_count>
<transaction_id><![CDATA[8911232246620200110152337851054]]></transaction_id>
<err_code><![CDATA[SUCCESS]]></err_code>
</xml>
<xml>
<openid><![CDATA[oaJiE1PwlwP_Tee0Z7uzrQFJeRqE]]></openid>
<trade_type><![CDATA[JSAPI]]></trade_type>
<cash_fee_type><![CDATA[CNY]]></cash_fee_type>
<nonce_str><![CDATA[20200116115914930]]></nonce_str>
<time_end><![CDATA[20200116115919]]></time_end>
<err_code_des><![CDATA[SUCCESS]]></err_code_des>
<return_code><![CDATA[SUCCESS]]></return_code>
<mch_id><![CDATA[128875980]]></mch_id>
<settlement_total_fee><![CDATA[101]]></settlement_total_fee>
<sign><![CDATA[B54319B2A47D9DC27F298DEC5A8B386A]]></sign>
<cash_fee><![CDATA[101]]></cash_fee>
<is_subscribe><![CDATA[Y]]></is_subscribe>
<return_msg><![CDATA[OK]]></return_msg>
<fee_type><![CDATA[CNY]]></fee_type>
<bank_type><![CDATA[CMC]]></bank_type>
<attach><![CDATA[pay-test]]></attach>
<device_info><![CDATA[sandbox]]></device_info>
<out_trade_no><![CDATA[wx20200116115914930]]></out_trade_no>
<result_code><![CDATA[SUCCESS]]></result_code>
<total_fee><![CDATA[101]]></total_fee>
<appid><![CDATA[wx28f9d88adc6a2f8c]]></appid>
<transaction_id><![CDATA[8766393522120200116115919172596]]></transaction_id>
<err_code><![CDATA[SUCCESS]]></err_code>
</xml>
依赖
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
<version>2.9.7</version>
</dependency>
配置XML转换器
@Configuration
public class MessageConverterConfig extends WebMvcConfigurationSupport {
@Override
public void configureMessageConverters(List<HttpMessageConverter<?>> converters) {
Jackson2ObjectMapperBuilder builder = Jackson2ObjectMapperBuilder.xml();
builder.indentOutput(true);
converters.add(new MappingJackson2XmlHttpMessageConverter(builder.build()));
}
}
参数模型
/**
* @author yezhou
* @version 1.0
* @date 2020/9/8 9:32
* @home http://www.appblog.cn
*/
@Data
@JacksonXmlRootElement(localName = "xml")
public class WechatPayNotifyDto {
@JacksonXmlProperty(localName = "mch_id")
private String mchId;
@JacksonXmlProperty(localName = "appid")
private String appid;
@JacksonXmlProperty(localName = "openid")
private String openid;
@JacksonXmlProperty(localName = "is_subscribe")
private String isSubscribe;
@JacksonXmlProperty(localName = "trade_type")
private String tradeType;
@JacksonXmlProperty(localName = "out_trade_no")
private String outTradeNo;
@JacksonXmlProperty(localName = "transaction_id")
private String transactionId;
@JacksonXmlProperty(localName = "fee_type")
private String feeType;
@JacksonXmlProperty(localName = "total_fee")
private String totalFee;
@JacksonXmlProperty(localName = "device_info")
private String deviceInfo;
@JacksonXmlProperty(localName = "time_end")
private String timeEnd;
@JacksonXmlProperty(localName = "return_code")
private String returnCode;
@JacksonXmlProperty(localName = "return_msg")
private String returnMsg;
@JacksonXmlProperty(localName = "result_code")
private String resultCode;
@JacksonXmlProperty(localName = "err_code")
private String errCode;
@JacksonXmlProperty(localName = "err_code_des")
private String errCodeDes;
@JacksonXmlProperty(localName = "cash_fee_type")
private String cashFeeType;
@JacksonXmlProperty(localName = "cash_fee")
private String cashFee;
@JacksonXmlProperty(localName = "settlement_total_fee")
private String settlementTotalFee;
@JacksonXmlProperty(localName = "nonce_str")
private String nonceStr;
@JacksonXmlProperty(localName = "sign")
private String sign;
@JacksonXmlProperty(localName = "bank_type")
private String bankType;
@JacksonXmlProperty(localName = "attach")
private String attach;
}
Controller层
/**
* @author yezhou
* @version 1.0
* @date 2020/9/8 9:31
* @home http://www.appblog.cn
*/
@Slf4j
@RestController
@RequestMapping("/")
public class XmlAcceptController {
@RequestMapping(value = "/payNotify", method = RequestMethod.POST, consumes = MediaType.APPLICATION_XML_VALUE, produces = MediaType.APPLICATION_XML_VALUE)
@ResponseBody
public String payCallback(@RequestBody WechatPayNotifyDto notifyDto) {
log.info(JSON.toJSONString(notifyDto));
return "success";
}
}
运行结果:
{
"appid":"wx28f9d88adc6a2f8c",
"attach":"pay-test",
"bankType":"CMC",
"cashFee":"101",
"cashFeeType":"CNY",
"deviceInfo":"sandbox",
"errCode":"SUCCESS",
"errCodeDes":"SUCCESS",
"feeType":"CNY",
"isSubscribe":"Y",
"mchId":"128875980",
"nonceStr":"20200116115914930",
"openid":"oaJiE1PwlwP_Tee0Z7uzrQFJeRqE",
"outTradeNo":"wx20200116115914930",
"resultCode":"SUCCESS",
"returnCode":"SUCCESS",
"returnMsg":"OK",
"settlementTotalFee":"101",
"sign":"B54319B2A47D9DC27F298DEC5A8B386A",
"timeEnd":"20200116115919",
"totalFee":"101",
"tradeType":"JSAPI",
"transactionId":"8766393522120200116115919172596"
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/04/01/spring-boot-receives-wechat-payment-notification-parameters/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

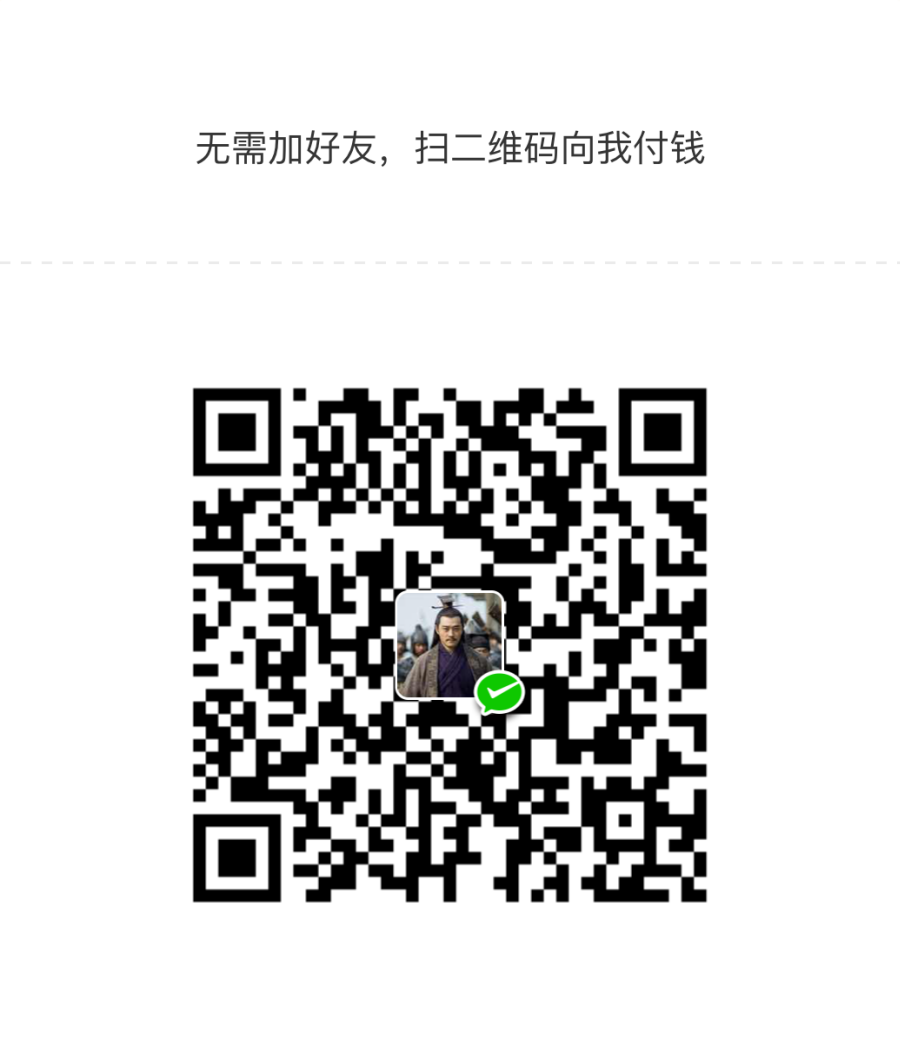
Spring Boot 接收微信支付通知参数
微信支付通知参数
首先明确微信支付通知参数是xml格式,示例如下:
<xml>
<openid><![CDATA[sandboxopenid]]></openid>
<tra……

文章目录
关闭
共有 0 条评论