Groovy代码示例 – list
list列表定义
def name = 'John'
def surname = 'Doe'
//字符串拼接
println 'Hello ' + name + ' ' + surname // Hello John Doe
//GString的使用
def name = 'John'
def surname = 'Doe'
//${varName} 变量占位符
println "Hello ${name} ${surname}" // Hello John Doe
println 'Hellow ${name} ${surname}' // Hellow ${name} ${surname}
列表(list)添加对象
groovy支持多种方式往列表list中添加对象。
- 1)我们可以使用
push
和<<
方法往列表list的尾部添加对象 - 2)支持像数组那样增加对象,如
cartoons[3]='Batman'
- 3)支持通过
plus
方法增加对象,你可以把这个方法当做是连接列表 - 4)支持通过
add/addAll
的方法将对象加入到列表中
def cartoons = ['Regular Show', 'The Amazing World of Gumball']
cartoons.push('Adventure Time')
cartoons[3] = 'Batman'
cartoons << 'Smurfs'
println cartoons // [Adventure Time, Regular Show, The Amazing World of Gumball, Batman, Smurfs]
cartoons.plus(4, 'Scooby Doo') //plus方法不会改变原列表数据
println cartoons // [Adventure Time, Regular Show, The Amazing World of Gumball, Batman, Smurfs]
def cartoonsWithPlus = cartoons.plus(2, 'Scooby Doo')
println cartoonsWithPlus // [Adventure Time, Regular Show, Scooby Doo, The Amazing World of Gumball, Batman, Smurfs]
cartoons.add(0, 'Johnny Test')
println cartoons // [Johnny Test, Adventure Time, Regular Show, The Amazing World of Gumball, Batman, Smurfs]
cartoons.addAll(2, ['Tom and Jerry', 'Uncle Grandpa']) // [Johnny Test, Adventure Time, Tom and Jerry, Uncle Grandpa, Regular Show, The Amazing World of Gumball, Batman, Smurfs]
println cartoons
列表list中删除对象
可以使用remove()
或者是pop()
方法从列表中删除对象。
def cartoons = ['Regular Show', 'The Amazing World of Gumball', 'Adventure Time']
def poppedElement = cartoons.pop()
println poppedElement // Adventure Time
println cartoons // [Regular Show, The Amazing World of Gumball]
cartoons.remove(0)
println cartoons // [The Amazing World of Gumball]
当使用pop方法的时候,总是删除列表的最后一个元素。你可以使用remove(index)删除指定位置的对象。
列表list的查找
很多时候我们需要对列表进行查找,以找到我们所需要的列表项。庆幸的是这在groovy中非常容易实现。
def cartoons = [
'Regular Show',
'The Amazing World of Gumball',
'Adventure Time',
'Uncle Grandpa',
'Batman',
'Scooby Doo'
]
def scoobyDoo = cartoons.find { it == 'Scooby Doo' }
println scoobyDoo // Scooby Doo
def cartoonNamesWithSizeGreaterThan12 = cartoons.findAll { it.size() > 12 }
println cartoonNamesWithSizeGreaterThan12 // [The Amazing World of Gumball, Adventure Time, Uncle Grandpa]
def cartoonNamesWithSizeGreaterThan15 = cartoons.findAll { cartoon -> cartoon.size() > 15 }
println cartoonNamesWithSizeGreaterThan15 // [The Amazing World of Gumball]
def cartoonsFoundWithRegex = cartoons.findAll { it =~ /an/ }
println cartoonsFoundWithRegex // [Uncle Grandpa, Batman]
def cartoonIndexList = cartoons.findIndexOf { it =~ /^A/ }
println cartoonIndexList // 2
def cartoonIndexListWithStartPoint = cartoons.findIndexOf(4) { it =~ /^A/ }
println cartoonIndexListWithStartPoint // -1
def cartoonLastIndex = cartoons.findLastIndexOf { it.size() > 10 }
println cartoonLastIndex // 3
通过find方法我们可以很容易的实现列表的查找,你可以直接使用it参数或是自定义参数别名,然后在闭包中定义需要查找的表达式。find方法会返回首个匹配的列表项,如果你想查找所有匹配的对象,你可以使用findAll
方法,该方法会返回一个符合查找表达式的结果列表。
有时候我们仅仅想查找匹配项的位置,那么你可以使用findIndexOf
,该方法还可以支持起始位置,当你指定了起始位置,方法将从该位置开始查找匹配的列表项。
如果你想查找符合条件的最后一个列表项,可以使用findLastIndexOf
方法获取最后一个匹配项的位置。
列表(list)的分组合并(collate)
示例如何使用groovy将完整的列表如何分割成为子列表。
def cartoons = [
'Regular Show',
'The Amazing World of Gumball',
'Adventure Time',
'Uncle Grandpa',
'Batman'
]
//两两合并
def cartoonsSplitListWithTwoCartoonEach = cartoons.collate(2)
println cartoonsSplitListWithTwoCartoonEach // [[Regular Show, The Amazing World of Gumball], [Adventure Time, Uncle Grandpa], [Batman]]
//三个一组合并
def cartoonsSplitListWithThreeCartoonEach = cartoons.collate(3)
println cartoonsSplitListWithThreeCartoonEach // [[Regular Show, The Amazing World of Gumball, Adventure Time], [Uncle Grandpa, Batman]]
//两个一组合并,舍弃孤立不成组的元素
def cartoonsSplitListWithoutRemainder = cartoons.collate(2, false)
println cartoonsSplitListWithoutRemainder // [[Regular Show, The Amazing World of Gumball], [Adventure Time, Uncle Grandpa]]
groovy只是将列表通过分组合并的方式分割成为多个子列表。collate的方法支持两个参数,第一个参数为子列表(分组)的大小,第二个参数表明剩下元素(未能分组的、孤立的)是否保留。
列表元素的个数统计(count)
如果想要知道列表的大小,可以通过size()方法获取。但是,如果你想统计列表中某个元素的出现次数,可以使用count方法获取。
def cartoons = [
'Regular Show',
'The Amazing World of Gumball',
'Adventure Time',
'Regular Show',
'Adventure Time',
'Adventure Time'
]
//计算列表的大小
def cartoonCount = cartoons.size()
println cartoonCount // 6
//统计'Adventure Time'出现次数
def advTimeCount = cartoons.count('Adventure Time')
println advTimeCount // 3
//统计'Regular Show'出现次数
def regShowCount = cartoons.count('Regular Show')
println regShowCount // 2
列表元素的操作
如果你相对列表中元素执行一些操作,groovy就为了提供了很方便的实现方式。举个例子,假如你想把列表中所有元素的字符转为大写或者仅仅让A开头的字符串大写。
def cartoons = [
'Regular Show',
'The Amazing World of Gumball',
'Adventure Time'
]
//定义只把A开头的字符串大写
def uc(String cartoon) {
if (cartoon =~ /^A/ ) cartoon.toUpperCase()
else cartoon
}
//全部大写
def cartoonsWithUpperCase = cartoons*.toUpperCase()
println cartoonsWithUpperCase // [REGULAR SHOW, THE AMAZING WORLD OF GUMBALL, ADVENTURE TIME]
//A开头的转大写
def cartoonStartsAToUppercase = cartoons.collect { cartoon -> self.uc(cartoon) }
println cartoonStartsAToUppercase // [Regular Show, The Amazing World of Gumball, ADVENTURE TIME]
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/05/06/groovy-code-example-list/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。

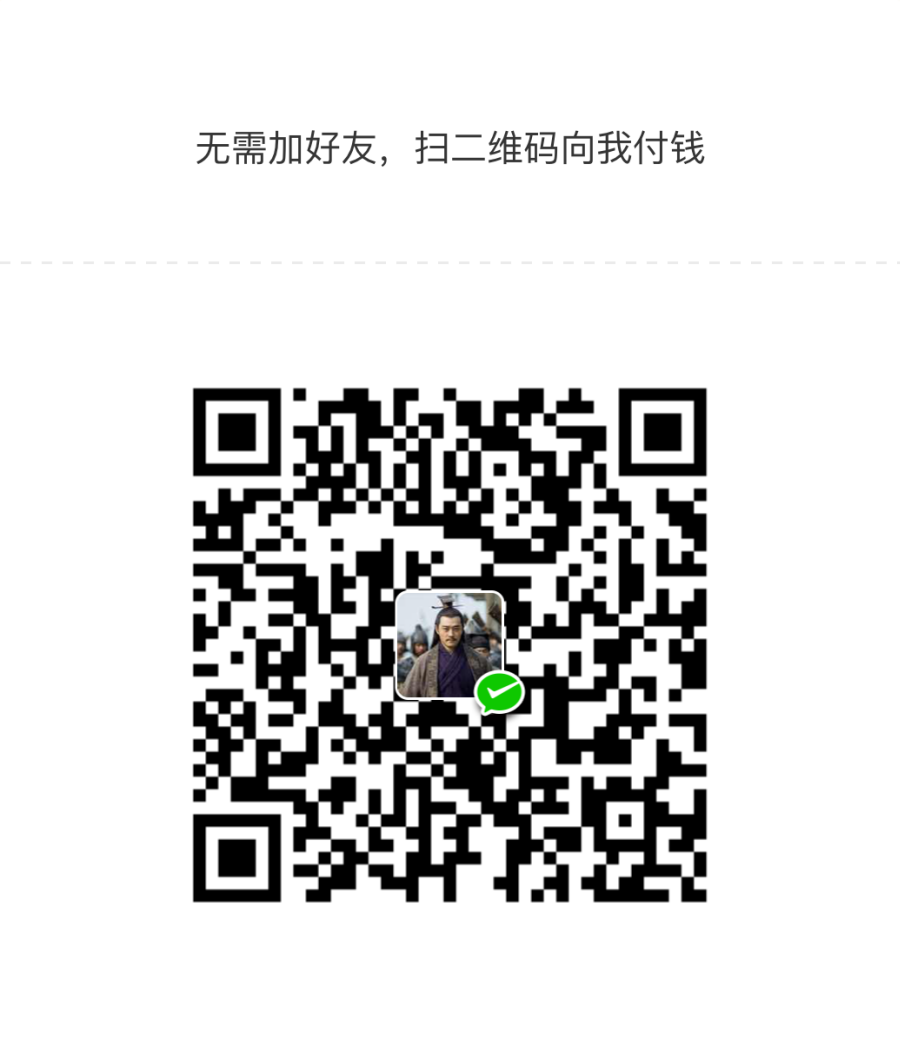

共有 0 条评论