Spring Cloud同步调用、异步调用、响应式调用
同步调用
@Configuration
public class WebConfig {
@Bean
@LoadBalanced
RestTemplate restTemplate(){
return new RestTemplate();
}
}
@Service
public class HelloService {
@Autowired
RestTemplate restTemplate;
@HystrixCommand(fallbackMethod = "helloFallBack")
public String hello() {
return restTemplate.getForEntity("http://HI-SERVICE/hello?name=Joe", String.class).getBody();
}
public String helloFallBack() {
return "error";
}
}
@RestController
public class HelloController {
@Autowired
HelloService helloService;
@RequestMapping(value = "/ribbon-hello", method = RequestMethod.GET)
public String hello() {
return helloService.hello();
}
}
异步调用
修改Service
@Service
public class HelloService {
@Autowired
RestTemplate restTemplate;
@HystrixCommand(fallbackMethod = "helloFallBack")
public String hello() {
return restTemplate.getForEntity("http://HI-SERVICE/hello?name=Joe", String.class).getBody();
}
@HystrixCommand(fallbackMethod = "helloFallBack")
public Future<String> helloAsync() {
return new AsyncResult<String>() {
@Override
public String invoke() {
return restTemplate.getForEntity("http://HI-SERVICE/hello?name=Joe", String.class).getBody();
}
};
}
public String helloFallBack() {
return "error";
}
}
修改Controller
@RestController
public class HelloController {
@Autowired
HelloService helloService;
@RequestMapping(value = "/ribbon-hello", method = RequestMethod.GET)
public String hello() {
return helloService.hello();
}
@RequestMapping(value = "/ribbon-hello-async", method = RequestMethod.GET)
public String helloAsync() throws ExecutionException, InterruptedException {
return helloService.helloAsync().get();
}
}
响应式调用
pom文件增加依赖
<!-- https://mvnrepository.com/artifact/io.reactivex.rxjava2/rxjava -->
<dependency>
<groupId>io.reactivex.rxjava2</groupId>
<artifactId>rxjava</artifactId>
<version>2.2.19</version>
</dependency>
修改Service
@Service
public class HelloService {
@Autowired
RestTemplate restTemplate;
@HystrixCommand(fallbackMethod = "helloFallBack")
public String hello() {
return restTemplate.getForEntity("http://HI-SERVICE/hello?name=Joe", String.class).getBody();
}
@HystrixCommand(fallbackMethod = "helloFallBack")
public Future<String> helloAsync() {
return new AsyncResult<String>() {
@Override
public String invoke() {
return restTemplate.getForEntity("http://HI-SERVICE/hello?name=Joe", String.class).getBody();
}
};
}
@HystrixCommand(fallbackMethod = "helloFallBack")
public Observable<String> helloResponse() {
return Observable.create(new Observable.OnSubscribe<String>() {
@Override
public void call(Subscriber<? super String> subscriber) {
String body = restTemplate.getForEntity("http://HI-SERVICE/hello?name=Joe", String.class).getBody();
subscriber.onNext(body);
subscriber.onCompleted();
}
});
}
public String helloFallBack() {
return "error";
}
}
修改Controller
@RequestMapping(value = "/ribbon-response", method = RequestMethod.GET)
public String helloResponse() throws ExecutionException, InterruptedException {
helloService.helloConsumerResponse().subscribe(new Subscriber<String>() {
@Override
public void onCompleted() {
System.out.println("完成");
}
@Override
public void onError(Throwable e) {
}
@Override
public void onNext(String s) {
System.out.println("接收到了:"+s);
}
});
return "ok";
}
版权声明:
作者:Joe.Ye
链接:https://www.appblog.cn/index.php/2023/03/26/spring-cloud-synchronous-call-asynchronous-call-responsive-call/
来源:APP全栈技术分享
文章版权归作者所有,未经允许请勿转载。
THE END
0
二维码
打赏
海报

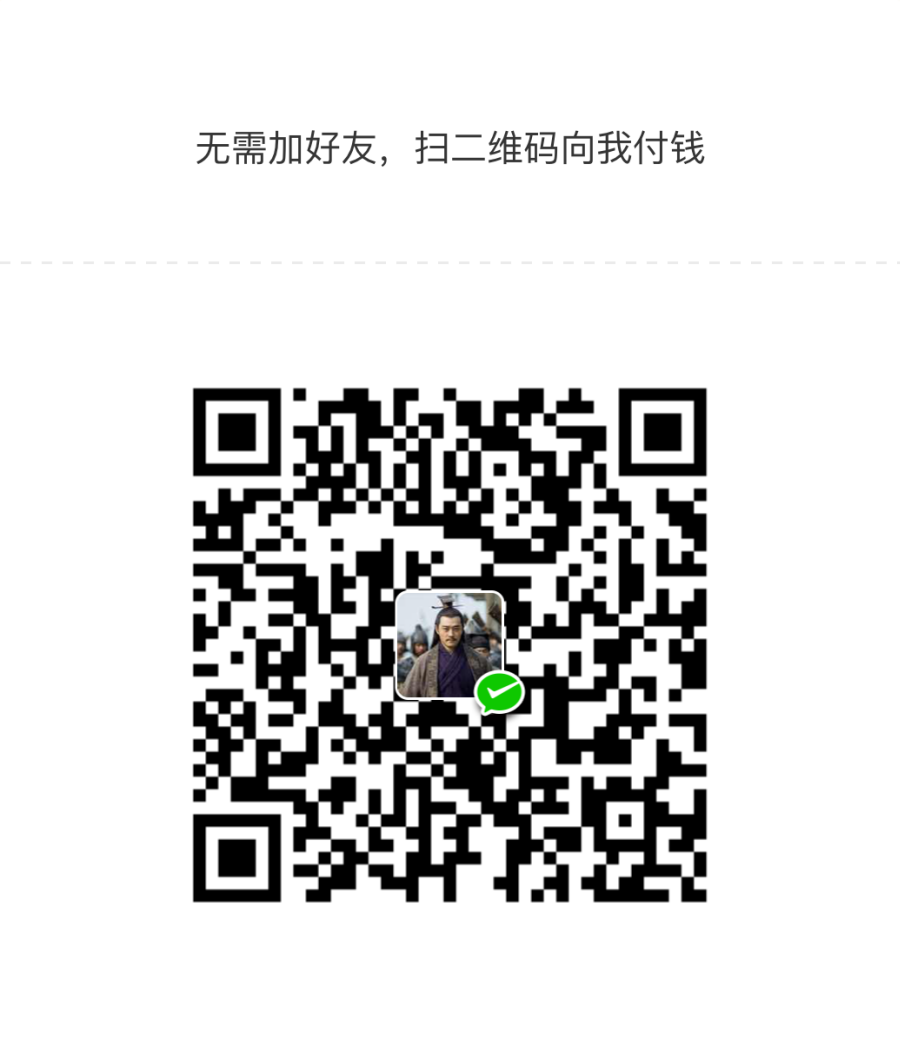
Spring Cloud同步调用、异步调用、响应式调用
同步调用
@Configuration
public class WebConfig {
@Bean
@LoadBalanced
RestTemplate restTemplate(){
return new RestTemplate();
……

文章目录
关闭
共有 0 条评论